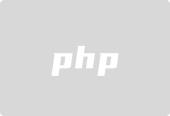
Guidelines for Writing STL-Compliant Containers
Introduction
Writing custom STL containers can be a challenging but rewarding task. To ensure that your container behaves seamlessly with the rest of the STL, it is crucial to adhere to established guidelines and best practices.
General Principles
-
Use Iterator Pattern: Iterators are the interface through which users access the elements of a container. Implement iterators that conform to the standard iterator categories and provide the necessary operations.
-
Follow the Strict Layout: Implement the container data structure according to the specified layout, including member function prototypes and the placement of member data and iterators.
-
Provide Essential Operations: Implement all essential operations required by a standard STL container, such as begin(), end(), size(), push_back(), erase(), and so on.
-
Handle Allocation: Use an allocator object for memory management. This allows the container to use user-defined memory management policies if needed.
-
Test Thoroughly: Utilize a class like tester to ensure that your container manages object lifecycles correctly and doesn't leak memory or introduce undefined behavior.
Specific Implementation Details
The provided code snippet outlines a basic structure for a sequence pseudo-container. Key implementation details include:
Iterator Class:
- Specify the iterator category (e.g., forward, random access).
- Implement necessary operations such as equality comparison, increment/decrement, addition/subtraction, and accessing the element.
Container Class:
- Specify the allocator type and value type.
- Declare iterator and const_iterator types.
- Define member functions for begin(), end(), push_front(), push_back(), and other standard operations.
Additional Notes:
- While most standard functions are technically optional, implementing them provides a comprehensive and fully featured container.
- Optionality is indicated using brackets [optional] in the function signatures.
- Swapping for containers is optionally defined and implemented externally.
- The tester class can help detect memory management issues and ensure proper object lifecycles within your container.
The above is the detailed content of How Can I Write a Custom STL-Compliant Container?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn