


After setting up JWT stateless authentication (available here), I wanted to understand what happens under Spring Security's abstractions by identifying key components and their interactions. To make this exploration more engaging, I reimplemented a minimal version in Go using the standard HTTP library. By breaking down three core flows - registration, token generation, and protected resource access - and rebuilding them in Go, I set out to map Spring Security's authentication patterns to simpler components.
This post focuses specifically on authentication flows - how the system verifies user identity - rather than authorization. We'll explore the flows with sequence diagrams that trace requests through different components in Spring Security's architecture.
Main Components
The system provides three endpoints:
- User Registration: Accepts username and password from new users
- Token Generation (Login): Creates a JWT token when users successfully log in with valid credentials
- Protected Access: Enables authenticated users to access protected resources using their token. The getAuthenticatedUser endpoint serves as an example, returning profile information for the authenticated token holder
In the following sections, I explain the core components involved in each flow, with a sequence diagram for each.
Registration Flow
A registration request containing username and password passes through the Spring Security filter chain, where minimal processing occurs since the registration endpoint was configured to not require authentication in SecurityConfiguration. The request then moves through Spring's DispatcherServlet, which routes it to the appropriate method in UserController based on the URL pattern. The request reaches UserController's register endpoint, where the user information is stored along with a hashed password.
Token Generation Flow
A login request containing username and password passes through the Spring Security filter chain, where minimal processing occurs as this endpoint is also configured to not require authentication in SecurityConfiguration. The request moves through Spring's DispatcherServlet to UserController's login endpoint, which delegates to AuthenticationManager. Using the configured beans defined in ApplicationConfiguration, AuthenticationManager verifies the provided credentials against stored ones. After successful authentication, the UserController uses JwtService to generate a JWT token containing the user's information and metadata like creation time, which is returned to the client for subsequent authenticated requests.
Protected Resource Access Flow
Successful Authentication Flow (200)
Failed Authentication Flow (401)
When a request containing a JWT token in its Authorization header arrives, it passes through the JwtAuthenticationFilter - a custom defined OncePerRequestFilter - which processes the token using JwtService. If valid, the filter retrieves the user via UserDetailsService configured in ApplicationConfiguration and sets the authentication in SecurityContextHolder. If the token is missing or invalid, the filter allows the request to continue without setting authentication.
Later in the chain, AuthorizationFilter checks if the request is properly authenticated via SecurityContextHolder. When it detects missing authentication, it throws an AccessDeniedException. This exception is caught by ExceptionTranslationFilter, which checks if the user is anonymous and delegates to the configured JwtAuthenticationEntryPoint in SecurityConfiguration to return a 401 Unauthorized response.
If all filters pass, the request reaches Spring's DispatcherServlet which routes it to the getAuthenticatedUser endpoint in UserController. This endpoint retrieves the authenticated user information from SecurityContextHolder that was populated during the filter chain process.
Note: Spring Security employs a rich ecosystem of filters and specialized components to handle various security concerns. To understand the core authentication flow, I only focused on the key players in JWT token validation and user authentication.
Go Implementation: Mapping Components
The Go implementation provides similar functionality through a simplified architecture that maps to key Spring Security components:
FilterChain
- Provides a minimal version of Spring Security's filter chain
- Processes filters sequentially for each request
- Uses a per-request chain instance (VirtualFilterChain) for thread safety
Dispatcher
- Maps to Spring's DispatcherServlet
- Routes requests to appropriate handlers after security filter processing
Authentication Context
- Uses Go's context package to store authentication state per request
- Maps to Spring's SecurityContextHolder
JwtFilter
- Direct equivalent to Spring's JwtAuthenticationFilter
- Extracts and validates JWT tokens
- Populates authentication context on successful validation
AuthenticationFilter
- Simplified version of Spring's AuthorizationFilter
- Solely focusing on authentication verification
- Checks authentication context and returns 401 if missing
JwtService
- Similar to Spring's JwtService
- Handles token generation and validation
- Uses same core JWT operations but with simpler configuration
Test Coverage
Both implementations include integration tests (auth_test.go and AuthTest.java) verifying key authentication scenarios:
Registration Flow
- Successful user registration with valid credentials
- Duplicate username registration attempt
Login Flow
- Successful login with valid credentials
- Login attempt with non-existent username
- Login attempt with incorrect password
Protected Resource Access
- Successful access with valid token
- Access attempt without auth header
- Access attempt with invalid token format
- Access attempt with expired token
- Access attempt with valid token format but non-existent user
The Java implementation includes detailed comments explaining the flow of each test scenario through Spring Security's filter chain. These same flows are replicated in the Go implementation using equivalent components.
Journey Summary
I looked at Spring Security's JWT auth by breaking it down into flows and test cases. Then I mapped these patterns to Go components. Integration tests showed me how requests flow through Spring Security's filter chain and components. Building simple versions of these patterns helped me understand Spring Security's design. The tests proved both implementations handle authentication the same way. Through analyzing, testing, and rebuilding, I gained a deeper understanding of how Spring Security's authentication works.
The above is the detailed content of Understanding JWT Authentication: Spring Securitys Architecture and Go Implementation. For more information, please follow other related articles on the PHP Chinese website!
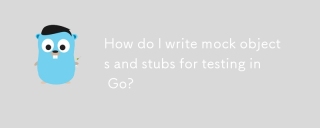
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
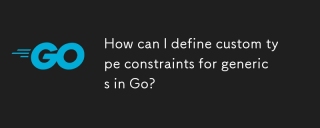
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
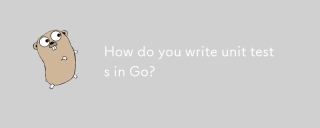
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
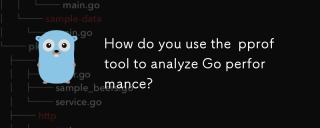
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
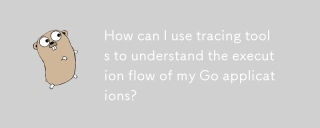
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
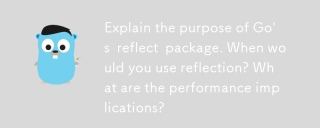
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
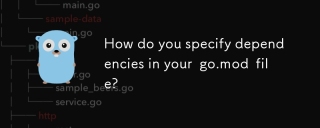
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
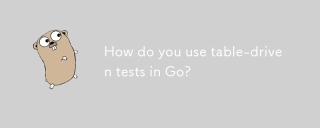
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
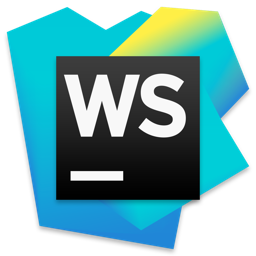
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
