


ConcurrentModificationException while Modifying an ArrayList During Iteration
The reported exception is a ConcurrentModificationException, originating from the attempt to modify an ArrayList, mElements, while iterating over it.
Inside an OnTouchEvent handler, there's a loop iterating over mElements using an Iterator to check for specific conditions:
for (Iterator<element> it = mElements.iterator(); it.hasNext();){ Element element = it.next(); // Check element's position and other conditions... if(element.cFlag){ mElements.add(new Element("crack",getResources(), (int)touchX,(int)touchY)); // ConcurrentModificationException occurs here element.cFlag = false; } }</element>
However, modifying the ArrayList (by adding a new element) while iterating over it using an Iterator can cause a ConcurrentModificationException.
Solution:
To avoid this exception, one option is to create a separate list to store elements that need to be added and append those to the main list after completing the iteration:
List<element> thingsToBeAdd = new ArrayList<element>(); for(Iterator<element> it = mElements.iterator(); it.hasNext();) { Element element = it.next(); // Check element's position and other conditions... if(element.cFlag){ // Store the new element in a separate list for later addition thingsToBeAdd.add(new Element("crack",getResources(), (int)touchX,(int)touchY)); element.cFlag = false; } } // Add all elements from the temporary list to the main list mElements.addAll(thingsToBeAdd );</element></element></element>
Alternative Approach:
Another approach is to use an enhanced for-each loop, which iterates over a copy of the list, preventing ConcurrentModificationException:
for (Element element : mElements) { // Check element's position and other conditions... if(element.cFlag){ mElements.add(new Element("crack",getResources(), (int)touchX,(int)touchY)); // No ConcurrentModificationException element.cFlag = false; } }
The above is the detailed content of How to Avoid ConcurrentModificationException When Modifying an ArrayList During Iteration?. For more information, please follow other related articles on the PHP Chinese website!
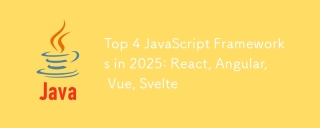
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
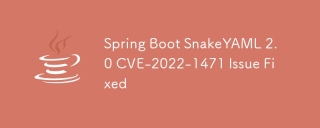
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
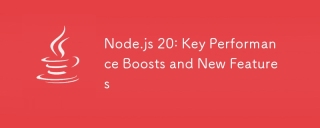
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
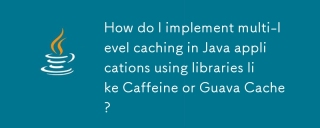
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
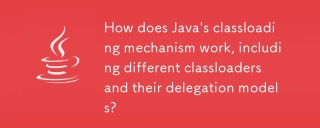
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
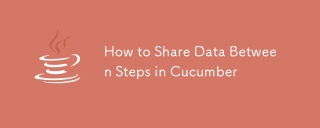
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
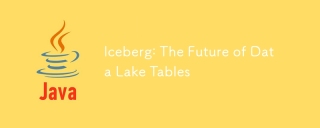
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
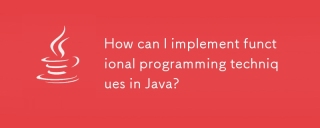
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
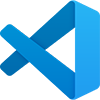
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
