


How Can I Efficiently Handle Non-Integer Constants in a C/C Switch Statement?
Switch with Non-Integer Constants in C/C
When working with non-POD (Plain Old Data) constants, it becomes necessary to select between different code paths based on the value of the constant. However, the traditional switch statement only accepts integer values. This presents a challenge for selecting between non-integer constants.
Traditional Approach: Nested if Statements
A simple way to handle this is to use a series of nested if statements, such as:
if( str == "foo" ) ... else if( str == "bar" ) ... else ...
While this is straightforward, it can become cumbersome and inefficient for large numbers of cases, as it has O(n) complexity where "n" is the number of cases.
Advanced Techniques
To achieve better efficiency, more advanced techniques can be employed. One approach involves using data structures like maps to represent strings as integers and then using a standard switch statement. However, this introduces additional coding complexity.
Compile-Time Magic: Macro and Template Magic
A unique approach is to use macro and template magic to generate an unrolled binary search at compile time. Libraries like fastmatch.h can provide a clean syntax for defining case matches:
NEWMATCH MATCH("asd") some c++ code MATCH("bqr") ... the buffer for the match is in _buf MATCH("zzz") ... user.YOURSTUFF /*ELSE optional */ ENDMATCH(xy_match)
This generates a function like xy_match(char *&_buf, T &user) that can be called with ease.
C 11 Updates: Lambdas and Initializer List
With C 11, lambdas and initializer lists provide a cleaner solution:
Switch("ger", { {"asdf", []{ printf("0\n"); }}, {"bde", []{ printf("1\n"); }}, {"ger", []{ printf("2\n"); }} }, [](const char *a, const char *b) { return strcmp(a, b) <p>This approach utilizes a binary search on a sorted list of case matches, providing efficient O(log n) complexity.</p><p><strong>Compile-Time Trie</strong></p><p>As a further advancement, compile-time tries can be leveraged to handle unsorted case-branches. This approach uses advanced C 11 metaprogramming to generate a search trie at compile time. Each trie node contains a switch statement to optimize code generation.</p><p>The implementation can be found on GitHub at smilingthax/cttrie.</p>
The above is the detailed content of How Can I Efficiently Handle Non-Integer Constants in a C/C Switch Statement?. For more information, please follow other related articles on the PHP Chinese website!
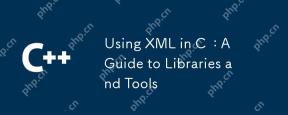
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
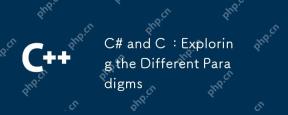
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
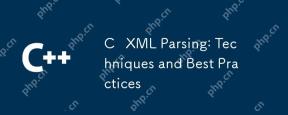
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
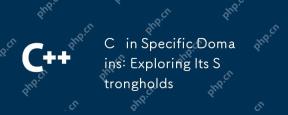
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
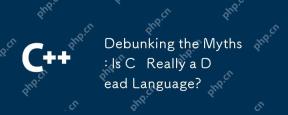
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
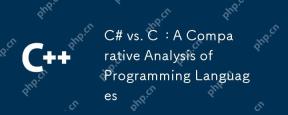
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
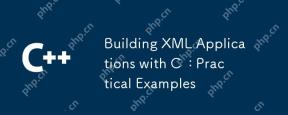
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
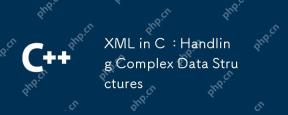
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
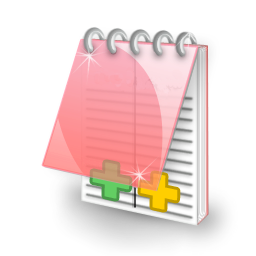
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
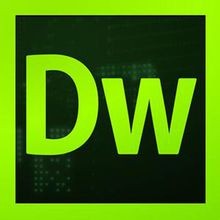
Dreamweaver CS6
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
