


How Can I Efficiently Replace Multiple Characters in a String with Their Escaped Versions?
Replacing Multiple Characters in a String
Problem:
How can I efficiently replace multiple specific characters in a string with their escaped versions (e.g., "&" with "&")?
Initial Attempt:
Loop through each desired replacement and apply it using the str.replace method:
strs = strs.replace('&', '\&') strs = strs.replace('#', '\#')
Alternative Approaches:
Option 1: Escape Loop
Create a loop that iterates over the characters you want to escape, checking if they exist in the string and escaping them if necessary:
def replace_multiple(text, chars): for c in chars: if c in text: text = text.replace(c, "\" + c) return text
This approach is simple and efficient, especially for a limited number of characters.
Option 2: Regular Expressions
Use regular expressions to find and replace multiple characters in the string:
import re text = re.sub('([&#])', r'\', text)
This method is more efficient if you need to replace a large number of characters.
Option 3: Lambda Function
Combine a lambda function with str.join to iterate over the string characters:
import string def escape_chars(string): return ''.join(r'\' + c if c in string.punctuation else c for c in string)
Option 4: Dict Replacements
Create a dictionary of desired replacements and use them to iterate over the string characters:
replacements = { "&": "\&", "#": "\#", "+": "\+", } text = "".join([replacements.get(c, c) for c in text])
Performance Comparison
The best approach for speed and readability will depend on the specific requirements and characteristics of the input string. Here are some timings for replacing various numbers of characters:
[Timings and code details are provided in the provided text]
The above is the detailed content of How Can I Efficiently Replace Multiple Characters in a String with Their Escaped Versions?. For more information, please follow other related articles on the PHP Chinese website!
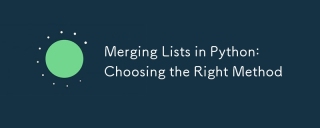
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
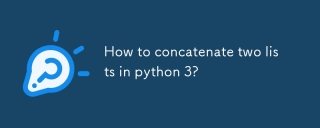
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
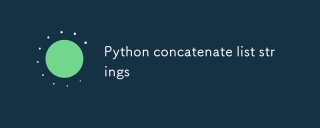
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
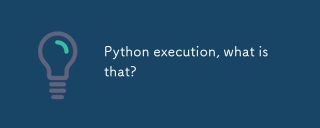
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
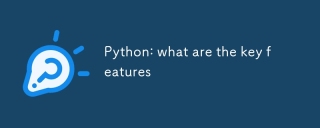
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
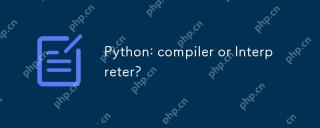
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
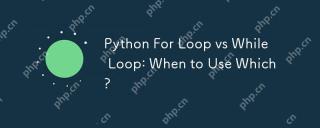
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
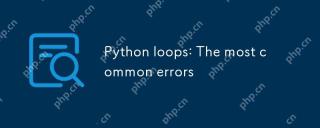
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
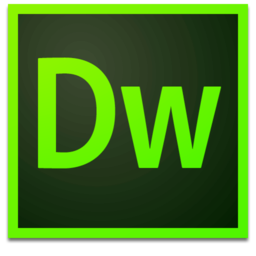
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
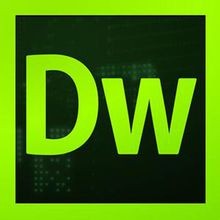
Dreamweaver CS6
Visual web development tools
