


Automating Data Cleanup in Firebase
To efficiently delete Firebase data that is older than two hours, consider the following approach:
Firebase Limitations:
Firebase does not offer queries with dynamic parameters like "two hours ago." However, it can execute queries for specific values, such as "after a particular timestamp."
Time-Based Deletion:
Implement a code snippet that executes periodically to delete data that is older than two hours at that time.
var ref = firebase.database().ref('/path/to/items/'); var now = Date.now(); var cutoff = now - 2 * 60 * 60 * 1000; var old = ref.orderByChild('timestamp').endAt(cutoff).limitToLast(1); var listener = old.on('child_added', function(snapshot) { snapshot.ref.remove(); });
Implementation Details:
- Use child_added instead of value and limitToLast(1) to handle deletions efficiently.
- As each child is deleted, Firebase will trigger child_added for the new "last" item until there are no more items after the cutoff point.
Cloud Functions for Firebase:
If you want this code to run periodically in the background, you can use Cloud Functions for Firebase:
exports.deleteOldItems = functions.database.ref('/path/to/items/{pushId}') .onWrite((change, context) => { var ref = change.after.ref.parent; var now = Date.now(); var cutoff = now - 2 * 60 * 60 * 1000; var oldItemsQuery = ref.orderByChild('timestamp').endAt(cutoff); return oldItemsQuery.once('value', function(snapshot) { var updates = {}; snapshot.forEach(function(child) { updates[child.key] = null; }); return ref.update(updates); }); });
Note:
- This function will trigger whenever data is written to /path/to/items, only deleting child nodes that meet the time criteria.
- The code is also available in the functions-samples repo for your convenience.
The above is the detailed content of How Can I Automate the Deletion of Data Older Than Two Hours in Firebase?. For more information, please follow other related articles on the PHP Chinese website!
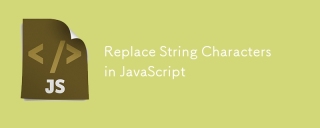
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
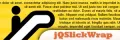
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
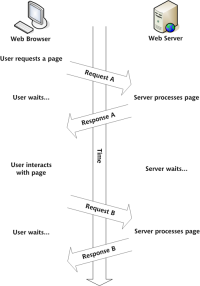
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
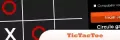
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
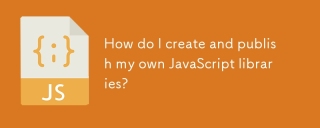
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
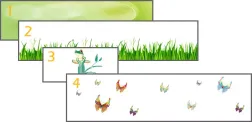
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
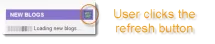
This tutorial demonstrates creating dynamic page boxes loaded via AJAX, enabling instant refresh without full page reloads. It leverages jQuery and JavaScript. Think of it as a custom Facebook-style content box loader. Key Concepts: AJAX and jQuery
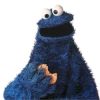
This JavaScript library leverages the window.name property to manage session data without relying on cookies. It offers a robust solution for storing and retrieving session variables across browsers. The library provides three core methods: Session


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
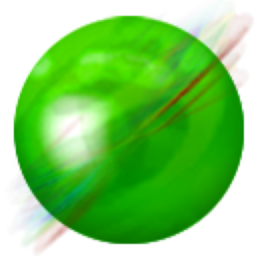
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
