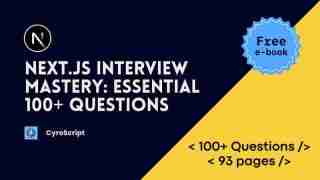
Next.js Interview Guide: 100 Questions and Answers to Succeed (Free)
Unlock your full potential in mastering Next.js with Next.js Interview Guide: 100 Questions and Answers to Succeed ?. Whether you're just starting out as a developer or you're an experienced professional looking to take your skills to the next level, this comprehensive e-book is designed to help you ace Next.js interviews and become a confident, job-ready developer. The guide covers a wide range of Next.js topics, ensuring you're well-prepared for any question that might come your way.This e-book explores key concepts like Server-Side Rendering (SSR) ?, Static Site Generation (SSG) ?, Incremental Static Regeneration (ISR) ⏳, App Router ?️, Data Fetching ?, and much more. Each topic is explained thoroughly, offering real-world examples and detailed answers to the most commonly asked interview questions. In addition to answering questions, the guide highlights best practices ✅ for optimizing your Next.js applications, improving performance ⚡, and ensuring scalability ?. With Next.js continuously evolving, we also dive deep into cutting-edge features like React 18, Concurrent Rendering, and Suspense ?. This makes sure you're always up-to-date with the latest advancements, equipping you with the knowledge that interviewers are looking for.What sets this guide apart is its practical approach. It doesn’t just cover theory but provides actionable insights that you can apply directly to your projects. Security ?, SEO optimization ?, and deployment practices ?️ are also explored in detail to ensure you're prepared for the full development lifecycle.Whether you're preparing for a technical interview at a top tech company or seeking to build more efficient, scalable applications, this guide will help you sharpen your Next.js skills and stand out from the competition. By the end of this book, you’ll be ready to tackle any Next.js interview question with confidence, from fundamental concepts to expert-level challenges.Equip yourself with the knowledge to excel as a Next.js developer ? and confidently step into your next career opportunity!
81. What are data fetching conventions in the App Router, and how do they differ from the Pages Router?
In Next.js with the App Router, data fetching follows new conventions that differ from the older Pages Router approach.
-
App Router Data Fetching:
- Server-side data fetching in the App Router is done using async functions in component-level code. Data fetching can occur directly within the component using useEffect or the new getServerSidePropslike function, but it integrates more closely with the component structure.
- Page-level layouts: You can define data fetching in the layout itself, making it easier to fetch data for multiple child pages and share that data across components.
- Parallel data fetching: The App Router allows multiple data fetching operations to be run in parallel (by returning an array of promises), making it more efficient than the Pages Router's sequential data fetching.
- Streaming and Suspense: The App Router leverages React Suspense and streaming, allowing pages to stream while other data is still being fetched, improving performance.
-
Pages Router Data Fetching:
- Data fetching in the Pages Router uses traditional methods such as getServerSideProps, getStaticProps, and getInitialProps. These functions are called at the page level and have a more static or server-bound approach.
- No nested layouts: In the Pages Router, data is typically fetched per page, and there’s no concept of layout-level data fetching or parallel data fetching like in the App Router.
- Synchronous rendering: Unlike the App Router, where data can be fetched asynchronously and streamed to the page, the Pages Router traditionally waits for all data to be fetched before rendering the page.
In summary:
- App Router introduces more flexible, parallelized, and streaming data fetching, with more integration at the component and layout levels.
- Pages Router relies on page-specific static and server-side data fetching methods.
82. What is the role of async components in Next.js, and why are they useful in the App Router?
In Next.js with the App Router, async components are used to enable server-side rendering (SSR) for components that need to fetch data asynchronously. These components are beneficial for scenarios where data is required before rendering the component but should be fetched from a server or external API. Using async components allows Next.js to optimize the rendering process by fetching the necessary data before the component is rendered on the server, improving performance and SEO.
Why are they useful in the App Router?
- Improved data fetching: async components allow for parallel data fetching, meaning multiple pieces of data can be fetched in parallel during SSR or in layouts, improving load times.
- Enhanced performance: Since data is fetched server-side and passed down to the component before rendering, it eliminates the need for waiting on data to load client-side, leading to a faster user experience.
- Integration with Suspense: The async nature of these components integrates well with React 18's Suspense, allowing components to "suspend" rendering until the data has been fetched, improving the user experience and making the app more scalable.
83. How does the App Router simplify data fetching with the new React 18 features?
The App Router simplifies data fetching with the introduction of React 18 features, primarily Suspense and Concurrent Rendering. These features allow Next.js to handle data fetching in a more efficient, flexible, and streamlined manner:
-
Suspense for Data Fetching:
- The App Router fully supports Suspense, which enables React components to suspend rendering while they wait for asynchronous operations (like data fetching) to complete. This makes it easier to handle loading states and asynchronous operations in the UI.
- Data fetching can be done asynchronously in the components themselves, and Suspense allows you to manage loading states for components waiting for data.
-
Concurrent Rendering:
- With React 18's concurrent rendering, Next.js can render components in the background and prioritize high-priority updates. This means data fetching can happen alongside rendering, and React can decide which components to render first based on importance, leading to faster page loads and better user experience.
- The App Router takes advantage of this by allowing multiple data fetching operations to run concurrently, making it easier to load complex pages that require data from different sources.
-
Streaming Data:
- The App Router also allows data to be streamed in parallel, meaning you can begin rendering parts of a page while waiting for other data to be fetched. This significantly reduces time-to-first-byte (TTFB) and allows users to see content faster.
-
Layouts:
- The App Router's layout system can handle data fetching for different levels of the application, so data fetching can be done at the layout level instead of each individual page, making it easier to manage and share data across multiple pages or components.
84. What is the new use hook, and how does it streamline async data handling in Next.js?
The use hook is a new feature introduced in React 18 and integrated into Next.js with the App Router. It is used to handle asynchronous data fetching directly in components, making it simpler and more declarative to fetch and handle async data in a functional component. The use hook is part of the Concurrent React features and is designed to simplify handling promises in React components.
How does it streamline async data handling in Next.js?
-
Simplicity: Instead of using useEffect and useState to manage the lifecycle of an asynchronous request, the use hook allows you to directly wait for promises to resolve and use the data once it is available.
-
Example:
import { use } from 'react'; function MyComponent() { const data = use(fetchData()); // fetchData is a promise return <div>{data}</div>; }
-
Automatic Suspense Integration: The use hook integrates seamlessly with Suspense, meaning that if a component is using the use hook to fetch data, React will automatically suspend the component until the data is available, showing a loading state in the meantime. This eliminates the need for manually handling loading states or using additional hooks.
Concurrent Rendering Support: The use hook takes advantage of React’s concurrent rendering capabilities, meaning that data fetching can happen in parallel with other rendering operations. This improves the efficiency of the app and makes it easier to manage async operations in complex applications.
Reduced Boilerplate: Traditionally, asynchronous data fetching in React involves managing loading, error, and success states manually using hooks like useState and useEffect. The use hook simplifies this by handling promise resolution directly inside components, reducing boilerplate code and making the codebase cleaner and more concise.
Summary:
- async components allow server-side fetching and optimization by loading data asynchronously before rendering, improving performance.
- React 18 features like Suspense and Concurrent Rendering, fully integrated with the App Router, simplify parallel data fetching and improve the user experience with faster rendering.
- The use hook streamlines async data handling by making it easier to fetch and use promises directly in components, reducing boilerplate and integrating seamlessly with Suspense.
84. How can you handle search parameters dynamically in the App Router?
In the App Router, handling dynamic search parameters can be done using the useSearchParams hook or by reading the query string in your server-side or page logic. The useSearchParams hook is provided by React to work with the query parameters dynamically within a component.
Example using useSearchParams:
import { use } from 'react'; function MyComponent() { const data = use(fetchData()); // fetchData is a promise return <div>{data}</div>; }
- Dynamic routing with query parameters: You can dynamically access query parameters and perform actions like fetching data based on the search terms or applying filters.
- URL manipulation: You can also modify query parameters using useSearchParams to manipulate the URL based on the user's interaction with the page, such as updating filters or search queries.
85. How does code-splitting work with the App Router?
In the App Router, code-splitting is handled automatically to optimize the delivery of your app's JavaScript. Next.js splits the JavaScript bundle based on routes and components, so only the code needed for the current page is loaded.
Key features of code-splitting in the App Router:
- Automatic splitting by route: When a user navigates to a specific route, only the JavaScript required for that route is loaded. This reduces the initial load time and improves performance.
- Server-side rendering (SSR) and Client-side rendering (CSR): The App Router optimizes code-splitting by rendering the initial page on the server (if using SSR) and then loading additional code on demand when switching between pages or routes on the client side.
- Suspense and Lazy Loading: The App Router can work with React Suspense to lazy-load components. This allows parts of the app to be loaded on demand when required, further optimizing performance.
Example of lazy loading components with code-splitting:
import { useSearchParams } from 'next/navigation'; export default function Page() { const searchParams = useSearchParams(); const searchTerm = searchParams.get('search') || ''; return ( <div> <h1 id="Search-Results-for-searchTerm">Search Results for: {searchTerm}</h1> {/* Render search results based on the searchTerm */} </div> ); }
This ensures that code-splitting is done dynamically, with components and routes loaded only when needed.
86. How does the App Router support Route Groups, and what are they used for?
In the App Router, Route Groups provide a way to organize your routes and apply layout, shared components, or middlewares to certain groups of pages without modifying the URL structure.
What are Route Groups used for?
- Grouping routes logically: Route groups allow developers to create logical divisions in the application while maintaining a clean URL structure.
- Shared layouts or components: You can apply shared layouts to multiple routes without impacting the URL. For example, a group of pages sharing a common sidebar or header can be grouped together under a single layout.
- Nested layouts: Route Groups support nested layouts for more granular control over page structures, such as a different layout for admin pages or public pages.
Example of Route Groups:
import dynamic from 'next/dynamic'; const DynamicComponent = dynamic(() => import('./DynamicComponent'), { loading: () => <p>Loading...</p>, }); export default function Page() { return ( <div> <h1 id="Page-with-dynamic-component">Page with dynamic component</h1> <dynamiccomponent></dynamiccomponent> </div> ); }
In this example:
- dashboard/, admin/, and public/ represent logical groupings of pages.
- You can assign layouts specific to each group, like an AdminLayout for the admin/ group or a PublicLayout for the public/ group.
How to create Route Groups:
Route groups are created using (), allowing you to structure your application without changing the actual route path:
import { use } from 'react'; function MyComponent() { const data = use(fetchData()); // fetchData is a promise return <div>{data}</div>; }
In this case, the URL path doesn't include (admin) or (public), but it allows you to keep routes organized under different sections.
Summary:
- Handling search parameters: You can dynamically access search parameters in the App Router using useSearchParams or directly accessing the query string in the server-side code.
- Code-splitting: The App Router automatically splits code based on routes, components, and pages, with support for SSR and CSR to improve performance.
- Route Groups: These help organize your routes logically while maintaining a clean URL structure, supporting shared layouts, and enabling complex routing configurations.
87. What are the best practices for organizing a large Next.js project with the App Router?
When organizing a large Next.js project with the App Router, it is important to focus on scalability, maintainability, and modularity. Here are some best practices for structuring and organizing a large project:
1. Use the app/ Directory for the App Router
With Next.js 13 and the App Router, use the app/ directory for routing instead of the traditional pages/ directory. This allows for more advanced routing features such as nested routes, layouts, and parallel routes, which are essential for larger projects.
-
Directory Structure:
- Use a clear and consistent naming convention for your directories and files. Structure your app based on features, such as having separate folders for different modules or sections of the app.
-
Example:
import { useSearchParams } from 'next/navigation'; export default function Page() { const searchParams = useSearchParams(); const searchTerm = searchParams.get('search') || ''; return ( <div> <h1 id="Search-Results-for-searchTerm">Search Results for: {searchTerm}</h1> {/* Render search results based on the searchTerm */} </div> ); }
2. Use Layouts for Common UI
Leverage layouts to avoid repetition and maintain consistent design across different pages or sections of your app. Layouts help share UI components like navigation bars, footers, or sidebars without repeating code.
-
Example:
import dynamic from 'next/dynamic'; const DynamicComponent = dynamic(() => import('./DynamicComponent'), { loading: () => <p>Loading...</p>, }); export default function Page() { return ( <div> <h1 id="Page-with-dynamic-component">Page with dynamic component</h1> <dynamiccomponent></dynamiccomponent> </div> ); }
- Split Features into Modules (Feature-based Structure)
A feature-based approach to organizing your project makes it easier to scale and maintain. Each feature can have its own directory with the necessary components, hooks, and utility functions.
-
Example:
import { use } from 'react'; function MyComponent() { const data = use(fetchData()); // fetchData is a promise return <div>{data}</div>; }
- Keep API Routes Organized
Use the app/api/ directory to handle API routes. Organize them based on the related feature or domain. This will help you keep your code modular and easier to manage.
-
Example:
import { useSearchParams } from 'next/navigation'; export default function Page() { const searchParams = useSearchParams(); const searchTerm = searchParams.get('search') || ''; return ( <div> <h1 id="Search-Results-for-searchTerm">Search Results for: {searchTerm}</h1> {/* Render search results based on the searchTerm */} </div> ); }
- Use TypeScript for Strong Typing
For larger projects, TypeScript is highly recommended because it improves code quality, provides autocompletion, and reduces runtime errors. Define types for props, states, and API responses to ensure better maintainability and scalability.
-
Example:
import dynamic from 'next/dynamic'; const DynamicComponent = dynamic(() => import('./DynamicComponent'), { loading: () => <p>Loading...</p>, }); export default function Page() { return ( <div> <h1 id="Page-with-dynamic-component">Page with dynamic component</h1> <dynamiccomponent></dynamiccomponent> </div> ); }
- Leverage API Middleware
For shared logic (e.g., authentication checks, logging, or caching), use middleware in the app/api/ directory to avoid duplicating logic across multiple API routes.
-
Example:
/app/ ├── dashboard/ │ └── page.js ├── admin/ │ └── page.js └── public/ └── page.js
- Optimize Data Fetching and Caching
Use incremental static regeneration (ISR) or server-side rendering (SSR) for pages that require real-time or dynamic data, and static generation (getStaticProps) for content that doesn’t change frequently. Combine this with caching and background regeneration for efficient data fetching.
-
Example:
/app/ ├── (admin)/ │ └── page.js // Admin group route ├── (public)/ │ └── page.js // Public group route
- Use Custom Hooks for Reusable Logic
Encapsulate reusable logic like data fetching, form handling, or state management into custom hooks. This helps you maintain clean and DRY code while avoiding repetition across components.
-
Example:
app/ ├── dashboard/ │ ├── page.js # Dashboard main page │ ├── settings/ # Nested route │ │ └── page.js ├── auth/ # Authentication-related pages │ ├── login/ │ └── register/ ├── user/ │ ├── profile/ │ └── account/ └── layout.js # Layout for the whole app
- Leverage Code Splitting and Lazy Loading
For large Next.js applications, use dynamic imports for code splitting and lazy loading components that are not required immediately. This reduces the initial bundle size and improves performance.
-
Example:
// app/layout.js export default function Layout({ children }) { return ( <div> <header></header> <main>{children}</main> <footer></footer> </div> ); }
- Implement Global State Management Carefully
In large Next.js applications, using React Context, Redux, or Zustand for state management can be crucial. However, be mindful of where the state is stored and avoid over-complicating the state management, especially if only small parts of the app need access to it.
-
Example using React Context:
app/ ├── dashboard/ │ ├── components/ │ ├── hooks/ │ ├── utils/ │ └── page.js ├── user/ │ ├── components/ │ ├── hooks/ │ └── page.js
Summary:
- Use the app/ directory for routing and layouts.
- Organize features into modules for easier maintenance.
- Keep API routes organized in the app/api/ directory.
- Leverage TypeScript for strong typing.
- Use middleware for shared logic across API routes.
- Optimize data fetching with SSR, SSG, and ISR.
- Utilize custom hooks for reusable logic.
- Apply code splitting with dynamic imports.
- Manage global state carefully with React Context or a state management library.
Following these best practices helps maintain clean, scalable, and high-performance code for large Next.js applications with the App Router.
88. What are the main steps to implement signup/login functionality in Next.js?
- Capture user credentials: Create a form that invokes a Server Action on submission.
- Validate form fields on the server: Use a schema validation library like Zod or Yup.
- Create a user account: Insert the user into the database or call an Auth Library’s API.
- Create a user session: Manage the user’s auth state using session management strategies.
89. How can you manage sessions in Next.js?
- Stateless sessions: Use libraries like iron-session or Jose to create and manage sessions.
- Database sessions: Store session data in a database and use cookies for optimistic auth checks.
90. What is the role of Middleware in Next.js authentication?
- Middleware can be used for initial checks to redirect users based on their authentication status. It should read the session from the cookie and avoid database checks to prevent performance issues.
The above is the detailed content of Next.js Interview Mastery: Essential Questions (Part 9). For more information, please follow other related articles on the PHP Chinese website!
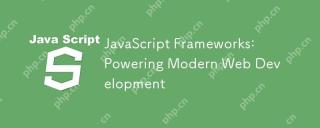
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
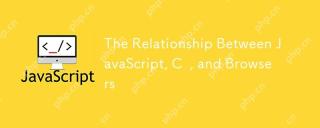
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
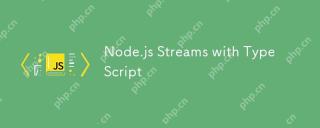
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
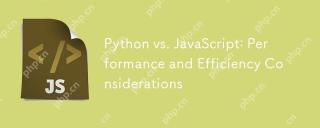
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
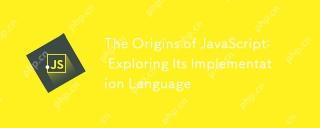
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
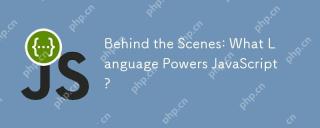
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
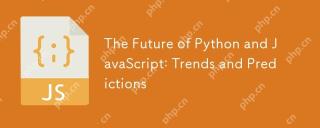
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
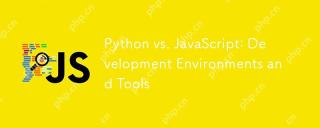
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
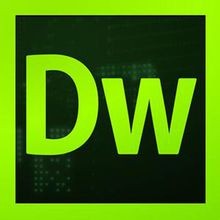
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
