Parsing Query Strings with JavaScript
In JavaScript, the window.location.search property contains the portion of the URL that begins with the question mark (?) and includes the query string parameters. This property can be used to access and manipulate these parameters.
However, JavaScript doesn't provide a built-in way to parse the query string into a key-value collection, as is commonly seen in ASP.NET. This has led to the development of custom solutions and libraries to address this need.
Custom Query String Parsing Function
Here's a custom function that you can use to parse the query string:
function getQueryString() { var result = {}, queryString = location.search.slice(1), re = /([^&=]+)=([^&=]*)/g, m; while ((m = re.exec(queryString))) { result[decodeURIComponent(m[1])] = decodeURIComponent(m[2]); } return result; }
This function iterates over the query string parameters, using a regular expression to capture the key and value of each parameter. It then decodes the URL-encoded strings and stores them in a JavaScript object.
Usage:
To use this function, you can simply call it and pass the window.location.search property as the argument:
var myParam = getQueryString()["myParam"];
This will assign the value of the myParam parameter to the myParam variable.
Note:
Keep in mind that this is a custom solution, and it's possible that major JavaScript libraries may provide their own implementations for parsing query strings. However, the provided function should suffice for most use cases.
The above is the detailed content of How Can I Parse Query Strings in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
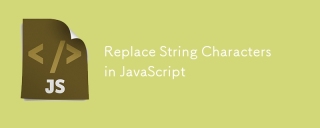
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
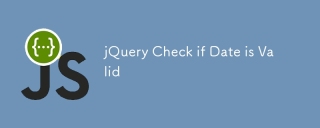
Simple JavaScript functions are used to check if a date is valid. function isValidDate(s) { var bits = s.split('/'); var d = new Date(bits[2] '/' bits[1] '/' bits[0]); return !!(d && (d.getMonth() 1) == bits[1] && d.getDate() == Number(bits[0])); } //test var
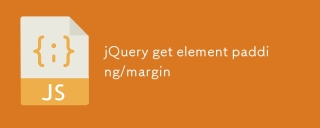
This article discusses how to use jQuery to obtain and set the inner margin and margin values of DOM elements, especially the specific locations of the outer margin and inner margins of the element. While it is possible to set the inner and outer margins of an element using CSS, getting accurate values can be tricky. // set up $("div.header").css("margin","10px"); $("div.header").css("padding","10px"); You might think this code is
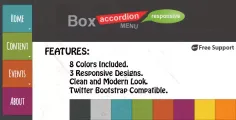
This article explores ten exceptional jQuery tabs and accordions. The key difference between tabs and accordions lies in how their content panels are displayed and hidden. Let's delve into these ten examples. Related articles: 10 jQuery Tab Plugins
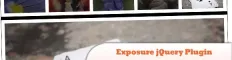
Discover ten exceptional jQuery plugins to elevate your website's dynamism and visual appeal! This curated collection offers diverse functionalities, from image animation to interactive galleries. Let's explore these powerful tools: Related Posts: 1
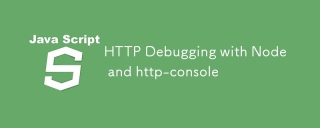
http-console is a Node module that gives you a command-line interface for executing HTTP commands. It’s great for debugging and seeing exactly what is going on with your HTTP requests, regardless of whether they’re made against a web server, web serv
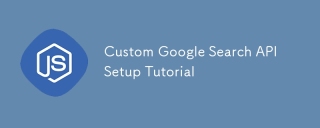
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
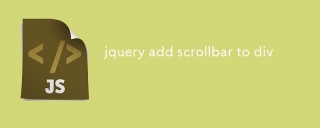
The following jQuery code snippet can be used to add scrollbars when the div content exceeds the container element area. (No demonstration, please copy it directly to Firebug) //D = document //W = window //$ = jQuery var contentArea = $(this), wintop = contentArea.scrollTop(), docheight = $(D).height(), winheight = $(W).height(), divheight = $('#c


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
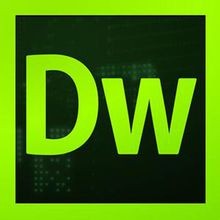
Dreamweaver CS6
Visual web development tools
