How to Force a DOM Redraw on Chrome for Mac
In web development, it's sometimes necessary to force the browser to redraw or refresh the DOM (Document Object Model). While there are various approaches that work in most browsers, Chrome for Mac poses a unique challenge.
The standard technique of modifying an unused CSS property, querying for an element dimension, and then reverting the change no longer works on Chrome for Mac. This behavior is due to optimizations that prevent redrawing when the offsetHeight property is retrieved.
To overcome this limitation, one can resort to a more invasive method:
$(el).css("border", "solid 1px transparent"); setTimeout(function() { $(el).css("border", "solid 0px transparent"); }, 1000);
While this method ensures a redraw, it also causes a visible jump in the element's position, which can be undesirable. A more subtle approach that guarantees a redraw is:
$('#parentOfElementToBeRedrawn').hide().show(0);
This hides and then immediately shows the parent element, forcing a reflow. Alternatively, in plain JavaScript:
document.getElementById('parentOfElementToBeRedrawn').style.display = 'none'; document.getElementById('parentOfElementToBeRedrawn').style.display = 'block';
For more fine-grained control over the redraw timing, a custom function can be defined:
var forceRedraw = function(element){ if (!element) { return; } var n = document.createTextNode(' '); var disp = element.style.display; // don't worry about previous display style element.appendChild(n); element.style.display = 'none'; setTimeout(function(){ element.style.display = disp; n.parentNode.removeChild(n); },20); // you can play with this timeout to make it as short as possible }
The above is the detailed content of How to Force a DOM Redraw in Chrome for Mac?. For more information, please follow other related articles on the PHP Chinese website!
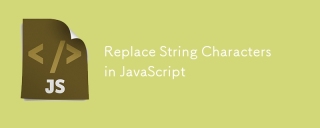
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
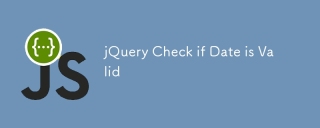
Simple JavaScript functions are used to check if a date is valid. function isValidDate(s) { var bits = s.split('/'); var d = new Date(bits[2] '/' bits[1] '/' bits[0]); return !!(d && (d.getMonth() 1) == bits[1] && d.getDate() == Number(bits[0])); } //test var
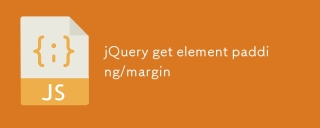
This article discusses how to use jQuery to obtain and set the inner margin and margin values of DOM elements, especially the specific locations of the outer margin and inner margins of the element. While it is possible to set the inner and outer margins of an element using CSS, getting accurate values can be tricky. // set up $("div.header").css("margin","10px"); $("div.header").css("padding","10px"); You might think this code is
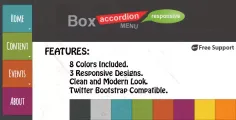
This article explores ten exceptional jQuery tabs and accordions. The key difference between tabs and accordions lies in how their content panels are displayed and hidden. Let's delve into these ten examples. Related articles: 10 jQuery Tab Plugins
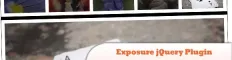
Discover ten exceptional jQuery plugins to elevate your website's dynamism and visual appeal! This curated collection offers diverse functionalities, from image animation to interactive galleries. Let's explore these powerful tools: Related Posts: 1
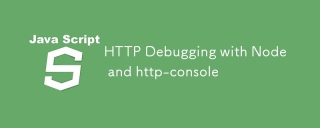
http-console is a Node module that gives you a command-line interface for executing HTTP commands. It’s great for debugging and seeing exactly what is going on with your HTTP requests, regardless of whether they’re made against a web server, web serv
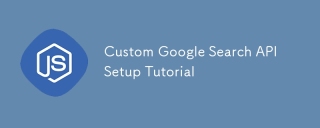
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
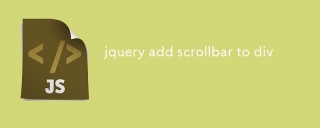
The following jQuery code snippet can be used to add scrollbars when the div content exceeds the container element area. (No demonstration, please copy it directly to Firebug) //D = document //W = window //$ = jQuery var contentArea = $(this), wintop = contentArea.scrollTop(), docheight = $(D).height(), winheight = $(W).height(), divheight = $('#c


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
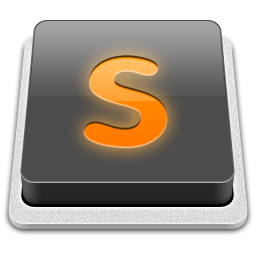
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
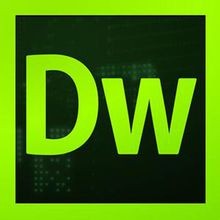
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
