Using Graphics2D to Overlay Text on a BufferedImage and Return a BufferedImage
In this example, we demonstrate how to overlay text on a BufferedImage using Graphics2D. We aim to provide a solution for rendering the text directly onto the image and returning the modified BufferedImage.
Initial Code and Discrepancy:
The provided code snippet attempted to achieve the text overlay but failed to make any visible changes to the image.
Issue:
The issue lies in how the drawString() method interprets the x and y coordinates. These coordinates denote the position of the baseline of the first character, not the top-left corner of the text. Therefore, depending on the font and the presence of descenders (characters like "g" or "p"), the text may end up outside the image bounds.
Solution:
To address this, we need to adjust the positioning of the text. Instead of using x and y directly, we first obtain the FontMetrics for the current Font object. The FontMetrics allows us to calculate the necessary offsets to position the text correctly.
Specifically, we compute the horizontal offset to ensure that the text is centered within the available width, and we adjust the vertical offset to avoid clipping the text.
Rewritten Code with Example:
import java.awt.Color; import java.awt.Dimension; import java.awt.Font; import java.awt.FontMetrics; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.image.BufferedImage; import java.io.IOException; import java.net.URL; import javax.imageio.ImageIO; import javax.swing.JFrame; import javax.swing.JPanel; public class TextOverlay extends JPanel { private BufferedImage image; public TextOverlay() { try { image = ImageIO.read(new URL( "http://cdn.sstatic.net/stackexchange/img/logos/so/so-logo.png")); } catch (IOException e) { e.printStackTrace(); } image = process(image); } @Override public Dimension getPreferredSize() { return new Dimension(image.getWidth(), image.getHeight()); } private BufferedImage process(BufferedImage old) { int w = old.getWidth() / 3; int h = old.getHeight() / 3; BufferedImage img = new BufferedImage( w, h,BufferedImage.TYPE_INT_ARGB); Graphics2D g2d = img.createGraphics(); g2d.drawImage(old, 0, 0, w, h, this); g2d.setPaint(Color.red); g2d.setFont(new Font("Serif", Font.BOLD, 20)); String s = "Hello, world!"; FontMetrics fm = g2d.getFontMetrics(); int x = img.getWidth() - fm.stringWidth(s) - 5; int y = fm.getHeight(); g2d.drawString(s, x, y); g2d.dispose(); return img; } @Override protected void paintComponent(Graphics g) { super.paintComponent(g); g.drawImage(image, 0, 0, null); } private static void create() { JFrame f = new JFrame(); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.add(new TextOverlay()); f.pack(); f.setVisible(true); } public static void main(String[] args) { javax.swing.SwingUtilities.invokeLater(new Runnable() { @Override public void run() { create(); } }); } }
In this modified code, we properly calculate the offsets based on the font metrics and render the text accordingly. As a result, the overlay text will be correctly positioned within the image.
The above is the detailed content of How to Correctly Overlay Text onto a BufferedImage using Java\'s Graphics2D?. For more information, please follow other related articles on the PHP Chinese website!
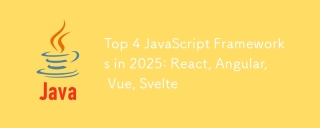
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
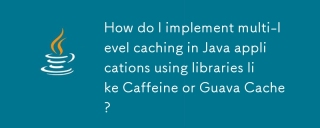
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
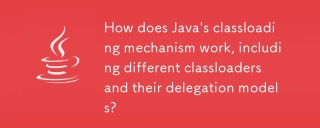
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
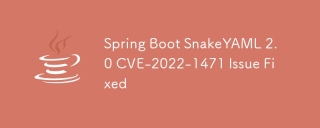
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
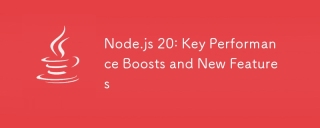
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
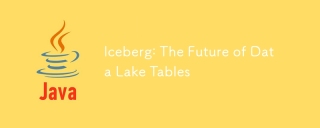
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
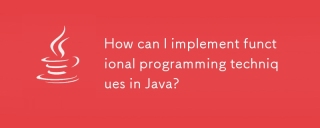
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
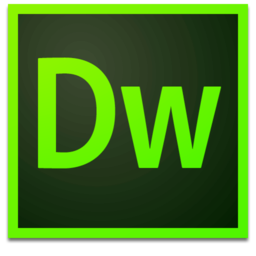
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
