Initializing Static Variables in PHP
When initializing static variables, you may encounter syntax errors like the one exemplified in the given code snippet. This is because PHP cannot parse complex expressions within the variable initializer.
Alternative Initialization Methods
To resolve this issue, consider using the following alternative methods:
- Separate Initialization: Define your static variable as usual, then initialize it using a separate statement outside of the class definition.
class Registration { static $dates; } Registration::$dates = array( 'start' => mktime(0, 0, 0, 7, 30, 2009), 'end' => mktime(0, 0, 0, 8, 2, 2009), 'close' => mktime(23, 59, 59, 7, 20, 2009), 'early' => mktime(0, 0, 0, 3, 19, 2009), );
- Initialization Function: Create a static function within the class to handle the initialization.
class Registration { private static $dates; static function init() { self::$dates = array( 'start' => mktime(0, 0, 0, 7, 30, 2009), 'end' => mktime(0, 0, 0, 8, 2, 2009), 'close' => mktime(23, 59, 59, 7, 20, 2009), 'early' => mktime(0, 0, 0, 3, 19, 2009), ); } // Call the init function to initialize the variable public function __construct() { static::init(); } }
PHP 5.6 Support
PHP 5.6 introduced limited support for non-trivial expressions in static variable initializers. However, it is recommended to use the aforementioned methods for clarity and compatibility with earlier versions of PHP.
The above is the detailed content of How Can I Properly Initialize Static Variables in PHP?. For more information, please follow other related articles on the PHP Chinese website!
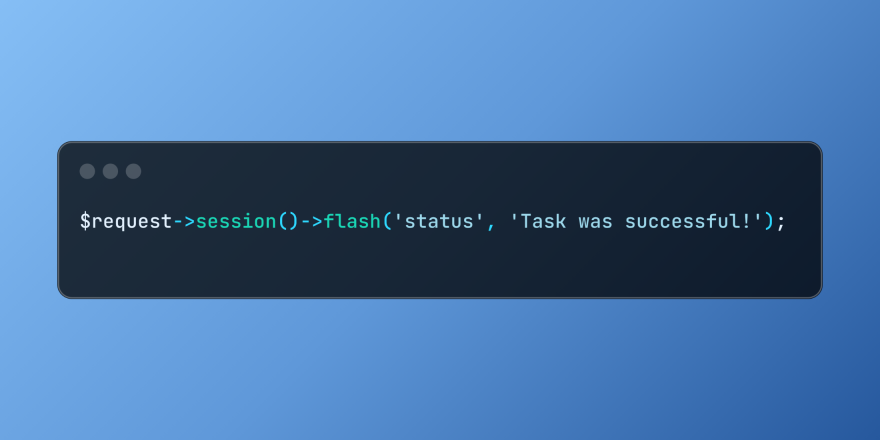
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
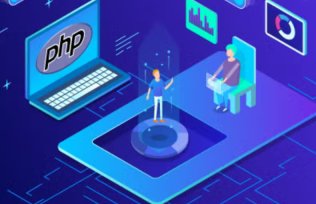
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
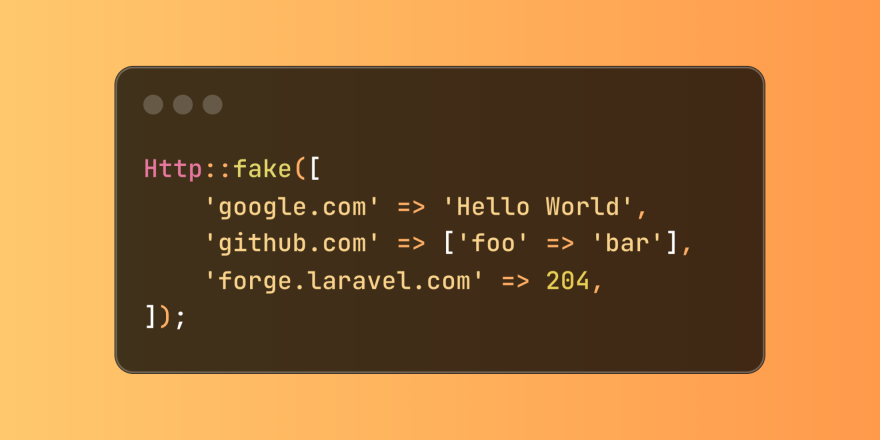
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
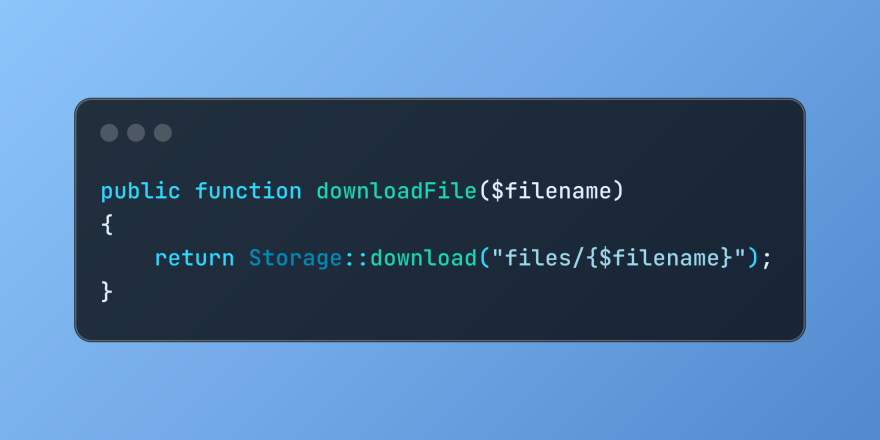
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
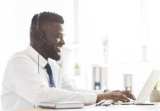
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
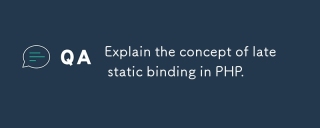
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
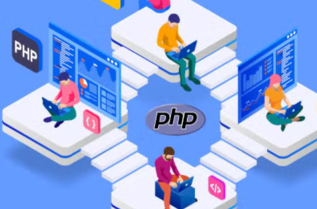
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
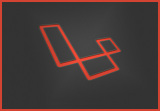
Laravel's service container and service providers are fundamental to its architecture. This article explores service containers, details service provider creation, registration, and demonstrates practical usage with examples. We'll begin with an ove


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
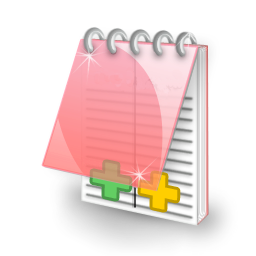
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
