Measuring Execution Time with Precision in C
Accurate execution time measurement is essential in optimizing code performance. In C , the clock() function provides a way to calculate elapsed time. However, it can return unreliable results with short code snippets.
Limitations of clock()
For small inputs or single-line statements, clock() often reports execution times of "0 seconds." This discrepancy arises because the clock() function has a granularity of milliseconds or nanoseconds, depending on the system. For execution durations shorter than this granularity, clock() rounds down to zero.
Solution: Using GetTimeMs64()
To overcome this limitation, consider using the utility function GetTimeMs64(). This function accurately measures the time elapsed since the Unix epoch, in milliseconds, and works on both Windows and Linux platforms.
The GetTimeMs64() function utilizes the system clock on Windows using GetSystemTimeAsFileTime(). On Linux, it employs gettimeofday() to retrieve the current time. It converts the obtained values to milliseconds and subtracts the offset from the Unix epoch to align with the Unix time standard.
Implementation
To use GetTimeMs64(), simply include the following code in your program:
#include <windows.h> // or #include <sys> #include <ctime> uint64 GetTimeMs64() { // Code from provided solution goes here }</ctime></sys></windows.h>
To calculate the execution time of a code snippet:
- Call GetTimeMs64() before executing the code.
- Execute the code.
- Call GetTimeMs64() again after executing the code.
- Subtract the first time from the second time to obtain the elapsed time in milliseconds.
Example Usage:
uint64 startTime = GetTimeMs64(); // Your code here uint64 endTime = GetTimeMs64(); cout <p>This method provides accurate execution time measurements even for small code snippets or short statements. It allows developers to optimize their code effectively and precisely gauge performance improvements.</p>
The above is the detailed content of How Can I Precisely Measure Code Execution Time in C ?. For more information, please follow other related articles on the PHP Chinese website!
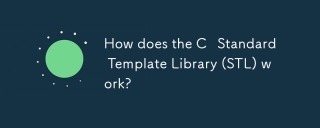
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
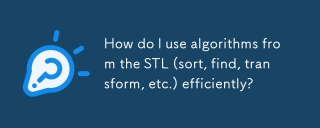
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
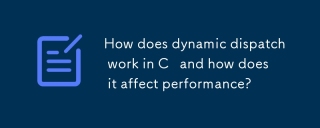
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
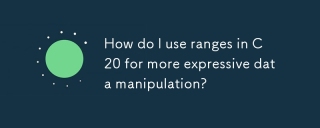
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
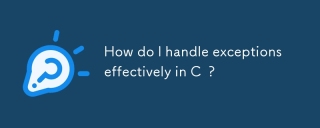
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
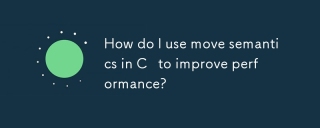
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
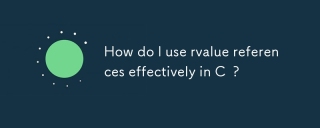
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
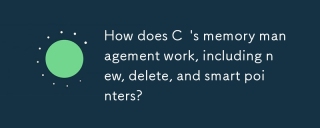
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
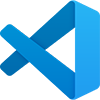
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
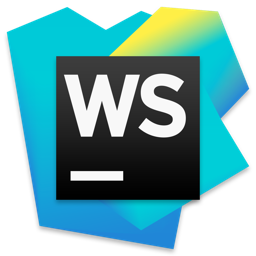
WebStorm Mac version
Useful JavaScript development tools
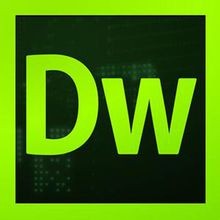
Dreamweaver CS6
Visual web development tools
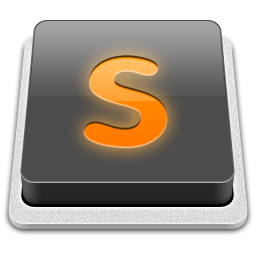
SublimeText3 Mac version
God-level code editing software (SublimeText3)
