Why Optimization Degrades This Function
In a university lecture on programming specialties, a lecturer presented a function that baffled students:
inline u64 Swap_64(u64 x) { u64 tmp; (*(u32*)&tmp) = Swap_32(*(((u32*)&x)+1)); (*(((u32*)&tmp)+1)) = Swap_32(*(u32*) &x); return tmp; }
Initially, the function operated smoothly, but upon enabling high optimization levels, it became inert. The reason behind this behavior lies in the concept of strict aliasing rules.
Strict Aliasing Violation
The provided code violates strict aliasing rules, which dictate that objects should only be accessed through compatible pointer types. In this case, the u32 and u64 pointers point to potentially overlapping memory, but the compiler assumes they represent distinct objects. This assumption allows it to optimize out assignments to the temporary variable tmp, rendering the function ineffective.
Why Optimization Occurs
The compiler is allowed to optimize code based on assumptions about pointer behavior. Since u32 and u64 are different types, the compiler assumes they do not point to the same memory and that the changes made through the u32 pointers will not affect tmp's value. This optimization leads to the observed behavior.
Solutions to Preserve Function Behavior
To prevent the code from being optimized out, the pointer types should match the accessed data types. One approach is to use a union to access the bits directly:
typedef union { uint32_t u32; uint16_t u16[2]; } U32; uint32_t swap_words(uint32_t arg) { U32 in; uint16_t lo; uint16_t hi; in.u32 = arg; hi = in.u16[0]; lo = in.u16[1]; in.u16[0] = lo; in.u16[1] = hi; return (in.u32); }
By using a union, we ensure that the pointers and data types are compatible, preventing the compiler from optimizing out the intended changes.
The above is the detailed content of Why Does Compiler Optimization Break This 64-bit Swap Function?. For more information, please follow other related articles on the PHP Chinese website!
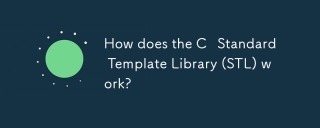
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
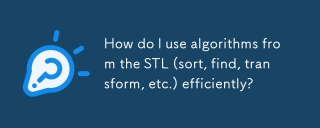
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
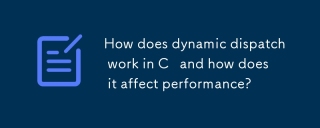
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
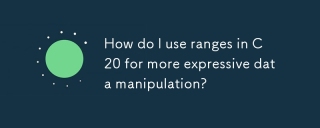
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
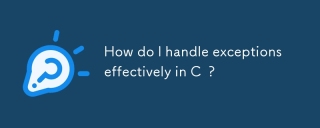
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
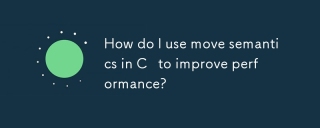
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
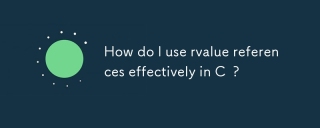
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
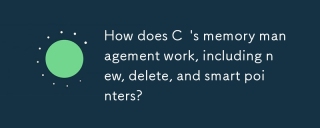
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
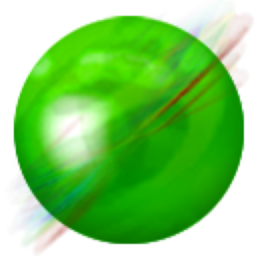
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
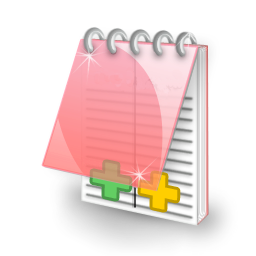
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
