


Pythonic Palindrome Check
In Python, checking for palindromes differs significantly from C-style for-loop approaches. Here's a more efficient Pythonic solution:
def is_palindrome(number): return str(number) == str(number)[::-1]
Advantages of the Pythonic Approach:
- Simplicity: The code is concise and easy to understand.
- Efficiency: Python optimizes for-loop operations, making this solution faster than C-style for loops.
- Readability: The Pythonic approach uses built-in string functions, making the code more readable.
Addressing Specific Questions:
- Pythonic Looping: To compare elements in a string, use slice notation to reverse it: string[::−1].
- For Loop in is_palindrome Function: You don't need a for loop since the Pythonic approach handles comparisons internally.
-
Resources for Beginner Python: For further guidance, consider:
- [Learn Python Faster](https://www.py4e.com)
- [Codecademy's Introduction to Python](https://www.codecademy.com/learn/learn-python)
-
Specific Algorithmic Tips:
- Check for special cases (zero or single-digit numbers).
- For numbers with odd length, divide by 2 and compare the first half with the reversed second half.
- For numbers with even length, divide by 2, and compare the two halves.
Example:
print(is_palindrome(121)) # True print(is_palindrome(234)) # False
By utilizing Python's built-in string functions and focusing on code clarity, you can efficiently determine if a value is a palindrome without the overhead of C-style for loops.
The above is the detailed content of How Can Python Efficiently Determine if a Number is a Palindrome?. For more information, please follow other related articles on the PHP Chinese website!
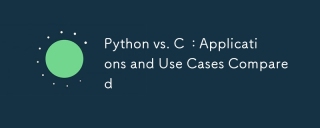
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
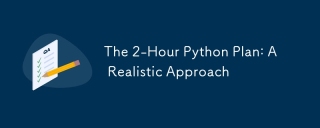
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
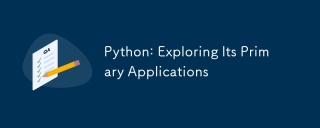
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
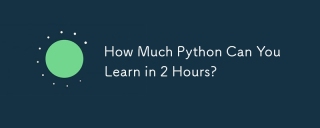
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
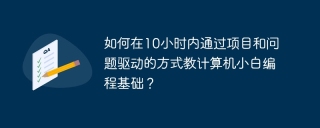
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
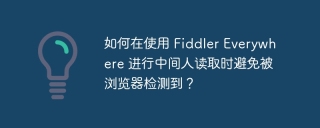
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
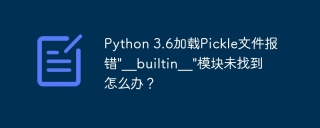
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...
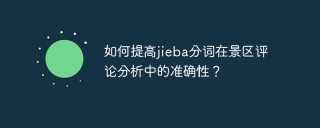
How to solve the problem of Jieba word segmentation in scenic spot comment analysis? When we are conducting scenic spot comments and analysis, we often use the jieba word segmentation tool to process the text...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
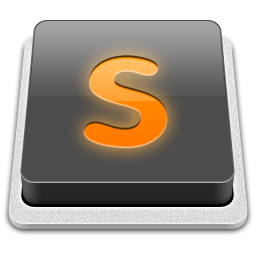
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
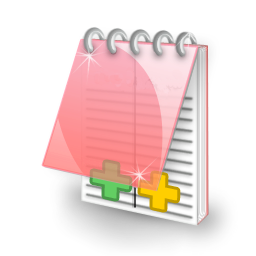
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function