Interrupting Tasks in an ExecutorService with Timeouts
Implementing a custom ExecutorService that handles task interruptions after a timeout can be challenging. However, the Java concurrency framework provides a convenient solution using the ScheduledExecutorService.
ScheduledExecutorService for Task Timeouts
A ScheduledExecutorService allows scheduling tasks for execution after a specified delay or at periodic intervals. By leveraging this feature, you can create a custom ExecutorService that monitors submitted tasks and interrupts them if they exceed a predefined timeout.
TimeoutThreadPoolExecutor Implementation
One approach to creating such an ExecutorService is the TimeoutThreadPoolExecutor class, which extends the standard ThreadPoolExecutor. It integrates a ScheduledExecutorService (timeoutExecutor) and a concurrent map (runningTasks) to track running tasks and schedule their interruptions.
Functioning of TimeoutThreadPoolExecutor
- beforeExecute: When a task is submitted, it's scheduled for interruption using timeoutExecutor. A ScheduledFuture is returned and stored in runningTasks, mapping the task to its scheduled interruption task.
- afterExecute: Once the task completes, its corresponding ScheduledFuture is removed from runningTasks and canceled, interrupting the task if it hasn't already completed.
- shutdown: Gracefully shuts down both ThreadPoolExecutor and ScheduledExecutorService.
- shutdownNow: Forcibly shuts down both ThreadPoolExecutor and ScheduledExecutorService.
Usage
To use this custom ExecutorService, instantiate a TimeoutThreadPoolExecutor with the desired parameters and start submitting tasks. The tasks will be interrupted after the specified timeout if they haven't completed.
Alternative Method Using Schedule
Another approach to interrupting long-running tasks in an ExecutorService is to use the schedule method provided by ScheduledExecutorService. You can submit two tasks: one that executes the actual task and another that cancels it after a specified timeout.
Code Example
ScheduledExecutorService executor = Executors.newScheduledThreadPool(2); Future handler = executor.submit(new Callable(){ ... }); executor.schedule(new Runnable(){ public void run(){ handler.cancel(); } }, 10000, TimeUnit.MILLISECONDS);
This method allows you to execute a task with a 10-second timeout, after which it will be canceled and interrupted.
The above is the detailed content of How Can I Implement Timed Task Interruption in a Java ExecutorService?. For more information, please follow other related articles on the PHP Chinese website!
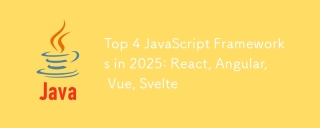
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
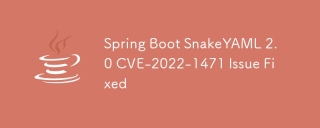
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
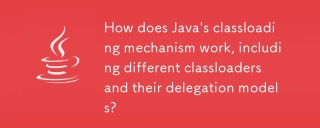
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
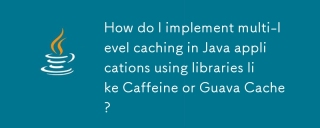
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
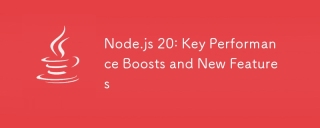
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
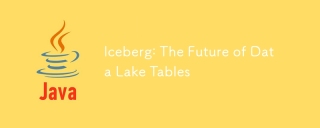
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
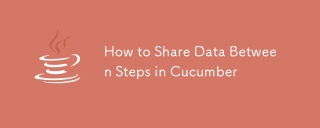
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
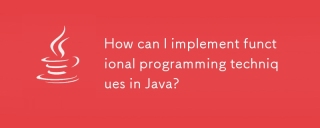
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
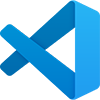
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
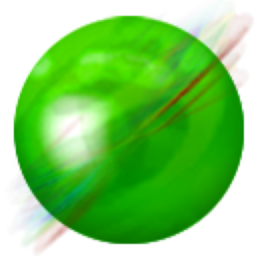
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
