


How to Unmarshal JSON into a Specific Struct Using an Interface Variable in Go?
How to Unmarshal JSON into a Specific Struct Using Interface Variables
Problem:
When unmarshaling JSON into an interface variable, the encoding/json package interprets it as a map. How can we instruct it to use a specific struct instead?
Explanation:
The JSON package requires a concrete type to target when unmarshaling. Passing an interface{} variable doesn't provide sufficient information, and the package defaults to map[string]interface{} for objects and []interface{} for arrays.
Solution:
There is no built-in way to tell json.Unmarshal to unmarshal into a concrete struct type using an interface variable. However, there is a workaround:
- Wrap a pointer to the desired struct in the interface variable.
func getFoo() interface{} { return &Foo{"bar"} }
- Pass the wrapped pointer to json.Unmarshal.
err := json.Unmarshal(jsonBytes, fooInterface)
- Access the unmarshaled data through the interface variable.
fmt.Printf("%T %+v", fooInterface, fooInterface)
Example:
The following code demonstrates the technique:
package main import ( "encoding/json" "fmt" ) type Foo struct { Bar string `json:"bar"` } func getFoo() interface{} { return &Foo{"bar"} } func main() { fooInterface := getFoo() myJSON := `{"bar":"This is the new value of bar"}` jsonBytes := []byte(myJSON) err := json.Unmarshal(jsonBytes, fooInterface) if err != nil { fmt.Println(err) } fmt.Printf("%T %+v", fooInterface, fooInterface) // prints: *main.Foo &{Bar:This is the new value of bar} }
The above is the detailed content of How to Unmarshal JSON into a Specific Struct Using an Interface Variable in Go?. For more information, please follow other related articles on the PHP Chinese website!
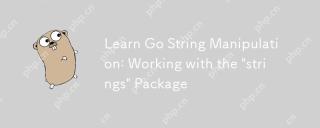
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
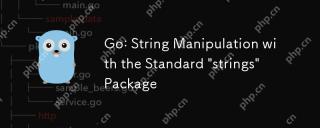
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
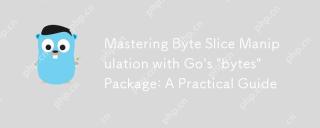
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
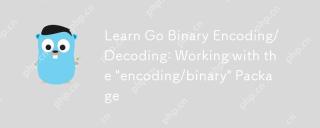
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
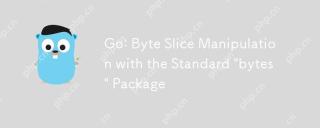
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
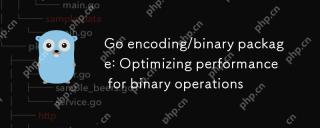
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
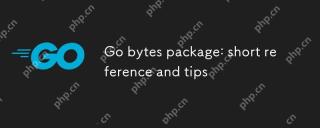
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
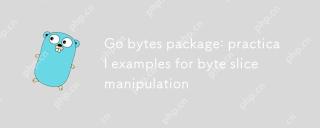
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
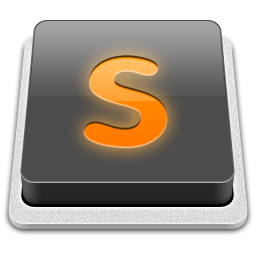
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
