


How to Reliably Handle Message Framing with net.Conn.Read in Go for Persistent TCP Sockets?
Proper Usage of net.Conn.Read for Persistent TCP Sockets
In Go, working with persistent TCP sockets involves establishing a connection and continuously reading incoming data. The net.Conn.Read function is responsible for retrieving data from the socket, but its behavior may not be immediately clear.
Understanding Message Framing in TCP
Unlike some other protocols, TCP does not inherently provide message framing. This means that it's up to the application to define a method of separating messages within the continuous data stream.
Traditional Message Framing with Header
In your previous C# experience, messages were prepended with a header containing the message size. This approach allows the receiving application to know the exact length of the subsequent message.
How Go Handles Message Handling
Go takes a different approach. By default, net.Conn.Read() does not have an inherent mechanism to determine the end of a message. The code snippet you provided in your question simply calls conn.Read() in a loop without considering message boundaries. This may work for certain scenarios, but it's not a reliable or scalable solution.
Solution: Custom Message Framing
To properly handle persistent TCP sockets, you need to implement custom message framing. This involves buffering the incoming data and parsing it according to your own defined protocol.
Example using bufio.Reader
One recommended approach is to use the built-in bufio.Reader to wrap your net.Conn. This wrapper provides additional functionality and makes it more efficient to read data.
import ( "bufio" "fmt" "io" "net" ) func main() { listener, err := net.Listen("tcp", ":8080") if err != nil { panic(err) } for { conn, err := listener.Accept() if err != nil { continue } go handleConnection(conn) } } func handleConnection(conn net.Conn) { defer conn.Close() r := bufio.NewReader(conn) for { size, err := r.ReadByte() if err != nil { return } buff := make([]byte, size) if _, err := io.ReadFull(r, buff); err != nil { return } fmt.Println("Received:", buff) } }
In this example, the handleConnection function first reads a single byte from the connection, representing the length of the subsequent message. It then creates a buffer and uses io.ReadFull to read the full message of that specified size. This allows the application to handle messages of varying lengths seamlessly.
The above is the detailed content of How to Reliably Handle Message Framing with net.Conn.Read in Go for Persistent TCP Sockets?. For more information, please follow other related articles on the PHP Chinese website!
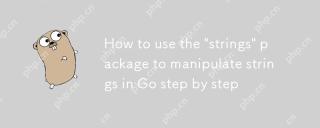
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
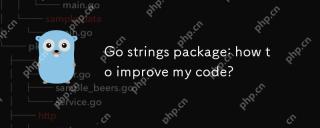
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
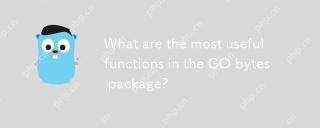
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
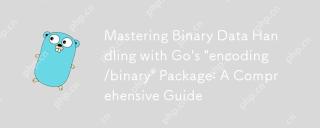
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
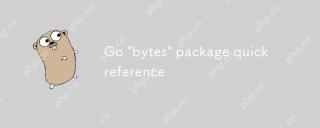
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
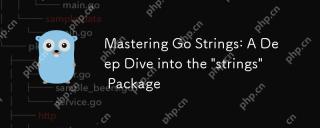
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
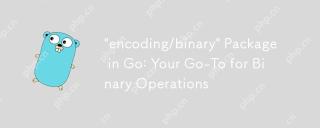
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
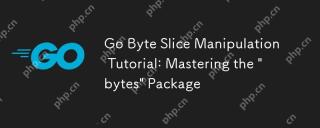
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
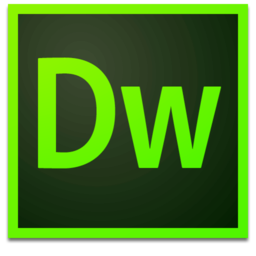
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
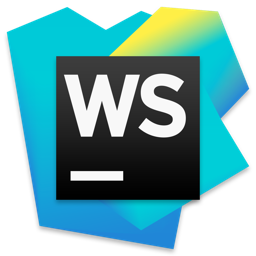
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
