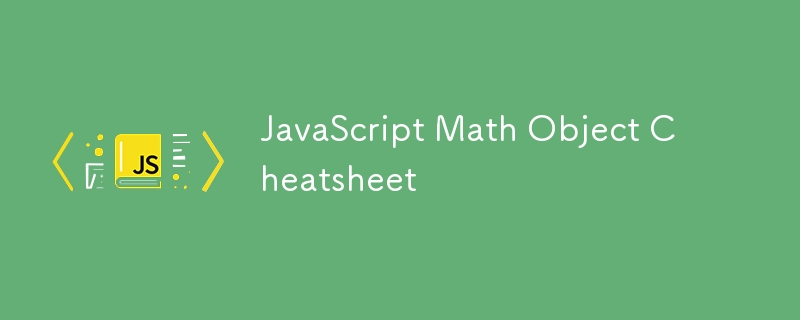
The Math object in JavaScript provides a set of properties and methods for performing mathematical tasks. Here's a comprehensive cheat sheet for the Math object.
Properties
The Math object has a set of constants:
Property |
Description |
Value (Approx.) |
Math.E |
Euler's number |
2.718 |
Math.LN2 |
Natural logarithm of 2 |
0.693 |
Math.LN10 |
Natural logarithm of 10 |
2.302 |
Math.LOG2E |
Base 2 logarithm of Math.E
|
1.442 |
Math.LOG10E |
Base 10 logarithm of Math.E
|
0.434 |
Math.PI |
Ratio of a circle's circumference to its diameter |
3.14159 |
Math.SQRT1_2 |
Square root of 1/2 |
0.707 |
Math.SQRT2 |
Square root of 2 |
1.414 |
Methods
1. Rounding Methods
Method |
Description |
Example |
Math.round(x) |
Rounds to the nearest integer |
Math.round(4.5) → 5
|
Math.floor(x) |
Rounds down to the nearest integer |
Math.floor(4.7) → 4
|
Math.ceil(x) |
Rounds up to the nearest integer |
Math.ceil(4.1) → 5
|
Math.trunc(x) |
Removes the decimal part (truncates) |
Math.trunc(4.9) → 4
|
2. Random Number Generation
Method |
Description |
Example |
Math.random() |
Generates a random number between 0 and 1 (exclusive) |
Math.random() → 0.53
|
Custom Random Int Generator |
Random integer between min and max
|
Math.floor(Math.random() * (max - min 1)) min |
3. Arithmetic Methods
Method |
Description |
Example |
Math.abs(x) |
Absolute value |
Math.abs(-7) → 7
|
Math.pow(x, y) |
Raises x to the power of y
|
Math.pow(2, 3) → 8
|
Math.sqrt(x) |
Square root of x
|
Math.sqrt(16) → 4
|
Math.cbrt(x) |
Cube root of x
|
Math.cbrt(27) → 3
|
Math.hypot(...values) |
Square root of the sum of squares of arguments |
Math.hypot(3, 4) → 5
|
4. Exponential and Logarithmic Methods
Method |
Description |
Example |
Math.exp(x) |
e^x |
Math.exp(1) → 2.718
|
Math.log(x) |
Natural logarithm (ln(x)) |
Math.log(10) → 2.302
|
Math.log2(x) |
Base 2 logarithm of x
|
Math.log2(8) → 3
|
Math.log10(x) |
Base 10 logarithm of x
|
Math.log10(100) → 2
|
5. Trigonometric Methods
Method |
Description |
Example |
Math.sin(x) |
Sine of x (x in radians) |
Math.sin(Math.PI / 2) → 1
|
Math.cos(x) |
Cosine of x (x in radians) |
Math.cos(0) → 1
|
Math.tan(x) |
Tangent of x (x in radians) |
Math.tan(Math.PI / 4) → 1
|
Math.asin(x) |
Arcsine of x (returns radians) |
Math.asin(1) → 1.57
|
Math.acos(x) |
Arccosine of x
|
Math.acos(1) → 0
|
Math.atan(x) |
Arctangent of x
|
Math.atan(1) → 0.785
|
Math.atan2(y, x) |
Arctangent of y / x
|
Math.atan2(1, 1) → 0.785
|
6. Min, Max, and Clamping
Method |
Description |
Example |
Math.max(...values) |
Returns the largest value |
Math.max(5, 10, 15) → 15
|
Math.min(...values) |
Returns the smallest value |
Math.min(5, 10, 15) → 5
|
Custom Clamping |
Restrict a value to a range |
Math.min(Math.max(x, min), max) |
7. Other Methods
Method |
Description |
Example |
Math.sign(x) |
Returns 1, -1, or 0 based on sign of x
|
Math.sign(-10) → -1
|
Math.fround(x) |
Nearest 32-bit floating-point number |
Math.fround(5.5) → 5.5
|
Math.clz32(x) |
Counts leading zero bits in 32-bit binary |
Math.clz32(1) → 31
|
Examples
Random Integer Between 1 and 100
const randomInt = Math.floor(Math.random() * 100) + 1;
console.log(randomInt);
Calculate Circle Area
const radius = 5;
const area = Math.PI * Math.pow(radius, 2);
console.log(area); // 78.54
Convert Degrees to Radians
const degrees = 90;
const radians = degrees * (Math.PI / 180);
console.log(radians); // 1.57
Find the Largest Number in an Array
const nums = [5, 3, 9, 1];
console.log(Math.max(...nums)); // 9
Extended Use Cases for the Math Object
The Math object has many practical applications. Here’s a list of common scenarios and examples to illustrate how to use it effectively.
1. Randomization
Generate a Random Integer Within a Range
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
console.log(getRandomInt(1, 10)); // Random number between 1 and 10
Shuffle an Array
function shuffleArray(arr) {
return arr.sort(() => Math.random() - 0.5);
}
console.log(shuffleArray([1, 2, 3, 4, 5])); // Shuffled array
Simulate a Dice Roll
function rollDice() {
return Math.floor(Math.random() * 6) + 1; // Random number between 1 and 6
}
console.log(rollDice());
2. Geometry and Shapes
Calculate the Area of a Circle
const radius = 5;
const area = Math.PI * Math.pow(radius, 2);
console.log(area); // 78.54
Calculate the Hypotenuse of a Triangle
const a = 3, b = 4;
const hypotenuse = Math.hypot(a, b);
console.log(hypotenuse); // 5
Convert Degrees to Radians
function degreesToRadians(degrees) {
return degrees * (Math.PI / 180);
}
console.log(degreesToRadians(90)); // 1.57
3. Finance and Business
Compound Interest Formula
function compoundInterest(principal, rate, time, n) {
return principal * Math.pow((1 + rate / n), n * time);
}
console.log(compoundInterest(1000, 0.05, 10, 12)); // 47.01
Rounding Currency Values
const amount = 19.56789;
const rounded = Math.round(amount * 100) / 100; // Round to 2 decimal places
console.log(rounded); // 19.57
Calculate Discounts
function calculateDiscount(price, discount) {
return Math.floor(price * (1 - discount / 100));
}
console.log(calculateDiscount(200, 15)); // 0
4. Games and Animation
Simulate a Coin Toss
function coinToss() {
return Math.random() < 0.5 ? 'Heads' : 'Tails';
}
console.log(coinToss());
Easing Functions for Smooth Animations
function easeOutQuad(t) {
return t * (2 - t); // Simple easing function
}
console.log(easeOutQuad(0.5)); // 0.75
Random Spawning Coordinates in a 2D Grid
function randomCoordinates(gridSize) {
const x = Math.floor(Math.random() * gridSize);
const y = Math.floor(Math.random() * gridSize);
return { x, y };
}
console.log(randomCoordinates(10)); // e.g., {x: 7, y: 2}
5. Data Analysis
Find the Maximum and Minimum in an Array
const scores = [85, 90, 78, 92, 88];
console.log(Math.max(...scores)); // 92
console.log(Math.min(...scores)); // 78
Normalize Data
function normalize(value, min, max) {
return (value - min) / (max - min);
}
console.log(normalize(75, 0, 100)); // 0.75
6. Physics and Engineering
Calculate Velocity After Free Fall
const gravity = 9.8; // m/s^2
const time = 3; // seconds
const velocity = gravity * time;
console.log(velocity); // 29.4 m/s
Period of a Pendulum
function pendulumPeriod(length) {
return 2 * Math.PI * Math.sqrt(length / 9.8);
}
console.log(pendulumPeriod(1)); // 2.006 seconds
7. Number Manipulation
Clamp a Number Within a Range
function clamp(value, min, max) {
return Math.min(Math.max(value, min), max);
}
console.log(clamp(15, 10, 20)); // 15
console.log(clamp(5, 10, 20)); // 10
Convert Negative Numbers to Positive
console.log(Math.abs(-42)); // 42
Find the Integer Part of a Number
console.log(Math.trunc(4.9)); // 4
console.log(Math.trunc(-4.9)); // -4
8. Problem-Solving
Check if a Number is a Power of 2
function isPowerOfTwo(n) {
return Math.log2(n) % 1 === 0;
}
console.log(isPowerOfTwo(8)); // true
console.log(isPowerOfTwo(10)); // false
Generate Fibonacci Numbers
function fibonacci(n) {
const phi = (1 + Math.sqrt(5)) / 2;
return Math.round((Math.pow(phi, n) - Math.pow(-phi, -n)) / Math.sqrt(5));
}
console.log(fibonacci(10)); // 55
9. Miscellaneous
Generate Random Colors (RGB)
function getRandomColor() {
const r = Math.floor(Math.random() * 256);
const g = Math.floor(Math.random() * 256);
const b = Math.floor(Math.random() * 256);
return `rgb(${r}, ${g}, ${b})`;
}
console.log(getRandomColor()); // e.g., rgb(123, 45, 67)
Calculate Age from Date of Birth
function calculateAge(birthYear) {
const currentYear = new Date().getFullYear();
return currentYear - birthYear;
}
console.log(calculateAge(1990)); // e.g., 34
The above is the detailed content of JavaScript Math Object Cheatsheet. For more information, please follow other related articles on the PHP Chinese website!