


How Can Java\'s `Scanner.useDelimiter()` Be Used with Regular Expressions to Split Input?
Understanding Scanner.useDelimiter with Regular Expressions
In Java's Scanner API, the useDelimiter() method allows you to specify a delimiter to split the input text into tokens. However, delimiters other than whitespace can be used, requiring the understanding of regular expressions (regex) patterns.
Regex Patterns in useDelimiter()
To use a delimiter with useDelimiter(), you need to provide a regex pattern. This pattern defines the characters or sequences that should separate the tokens.
For example, the code snippet below uses the delimiter "|,|rn" to split the input based on commas (",") or new lines (rn):
sc = new Scanner(new File(dataFile)); sc.useDelimiter(",|\r\n");
This means that the scanner will treat commas and new lines as separators, splitting the input into tokens.
Examples
To illustrate how useDelimiter() works with regex patterns, consider the following example:
String input = "1 fish 2 fish red fish blue fish"; // Use "\s*fish\s*" as the delimiter to split the input Scanner s = new Scanner(input).useDelimiter("\s*fish\s*"); System.out.println(s.nextInt()); // prints: 1 System.out.println(s.nextInt()); // prints: 2 System.out.println(s.next()); // prints: red System.out.println(s.next()); // prints: blue
Here, the delimiter "sfishs" matches 0 or more repetitions of whitespace characters ("s*") followed by the word "fish" and again 0 or more repetitions of whitespace. As a result, the input is split into tokens based on the delimiter, and the scanner can extract the desired values.
Additional Resources
For more information on useDelimiter() and regex patterns, refer to the following resources:
- Scanner API: https://docs.oracle.com/javase/7/docs/api/java/util/Scanner.html
- Regex Tutorial: https://www.vogella.com/tutorials/JavaRegularExpressions/article.html
- Regex Quick Reference: https://www.regular-expressions.info/quickref.html
The above is the detailed content of How Can Java\'s `Scanner.useDelimiter()` Be Used with Regular Expressions to Split Input?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
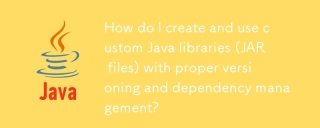
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
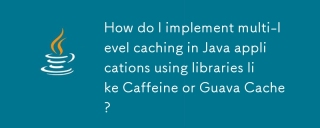
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
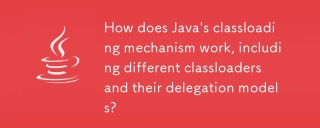
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
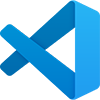
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
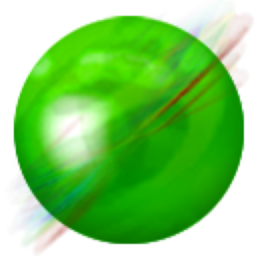
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
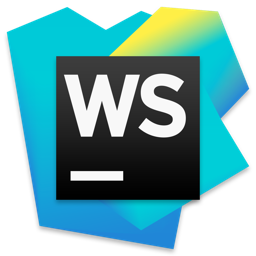
WebStorm Mac version
Useful JavaScript development tools