When working with objects and arrays in JavaScript, creating copies of data structures is a common task. However, developers often face challenges when deciding between a shallow copy and a deep copy. Misunderstanding the differences can lead to unintended side effects in your code. Let’s dive into these concepts, their differences, and when to use each.
? Download eBook - JavaScript: from ES2015 to ES2023
What Is a Shallow Copy?
A shallow copy creates a new object with copies of the top-level properties of the original object. For properties that are primitives (e.g., numbers, strings, booleans), the value itself is copied. However, for properties that are objects (like arrays or nested objects), only the reference is copied—not the actual data.
This means that while the new object has its own copy of top-level properties, the nested objects or arrays remain shared between the original and the copy.
Example of a Shallow Copy
const original = { name: "Alice", details: { age: 25, city: "Wonderland" } }; // Shallow copy const shallowCopy = { ...original }; // Modify the nested object in the shallow copy shallowCopy.details.city = "Looking Glass"; // Original object is also affected console.log(original.details.city); // Output: "Looking Glass"
How to Create a Shallow Copy
- Using the Spread Operator (...):
const shallowCopy = { ...originalObject };
- Using Object.assign():
const shallowCopy = Object.assign({}, originalObject);
While these methods are fast and easy, they are not suitable for deeply nested objects.
What Is a Deep Copy?
A deep copy duplicates every property and sub-property of the original object. This ensures that the copy is completely independent of the original, and changes to the copy do not affect the original object.
Deep copying is essential when dealing with complex data structures like nested objects or arrays, particularly in scenarios where data integrity is critical.
Example of a Deep Copy
const original = { name: "Alice", details: { age: 25, city: "Wonderland" } }; // Shallow copy const shallowCopy = { ...original }; // Modify the nested object in the shallow copy shallowCopy.details.city = "Looking Glass"; // Original object is also affected console.log(original.details.city); // Output: "Looking Glass"
How to Create a Deep Copy
-
Using JSON.stringify() and JSON.parse():
- Converts the object into a JSON string and then parses it back into a new object.
- Limitations:
- Cannot handle circular references.
- Ignores properties like functions, undefined, or Symbol.
const shallowCopy = { ...originalObject };
-
Using Libraries:
- Libraries like Lodash provide robust deep cloning methods.
const shallowCopy = Object.assign({}, originalObject);
-
Custom Recursive Function:
- For full control, you can write a recursive function to clone nested objects.
Comparing Shallow Copy and Deep Copy
Feature | Shallow Copy | Deep Copy | |||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
Copies only top-level properties. | Copies all levels, including nested data. | |||||||||||||||
References | Nested references are shared. | Nested references are independent. | |||||||||||||||
Performance | Faster and lightweight. | Slower due to recursive operations. | |||||||||||||||
Use Cases | Flat or minimally nested objects. | Deeply nested objects or immutable structures. |
When to Use Shallow Copy
- Flat Objects: When dealing with simple objects without nested properties.
- Performance: When speed is crucial, and you don’t need to handle deeply nested data.
- Temporary Changes: When you intend to modify top-level properties but share nested data.
Example Use Case
Copying a user’s settings object to make quick adjustments:
const original = { name: "Alice", details: { age: 25, city: "Wonderland" } }; // Shallow copy const shallowCopy = { ...original }; // Modify the nested object in the shallow copy shallowCopy.details.city = "Looking Glass"; // Original object is also affected console.log(original.details.city); // Output: "Looking Glass"
When to Use Deep Copy
- Complex Structures: For objects with multiple levels of nesting.
- Avoiding Side Effects: When you need to ensure that changes in the copy don’t affect the original.
- State Management: In frameworks like React or Redux, where immutability is critical.
Example Use Case
Duplicating the state of a game or application:
const shallowCopy = { ...originalObject };
Common Mistakes and Pitfalls
-
Assuming Shallow Copy Is Always Sufficient:
- Developers often mistakenly use shallow copy methods for nested objects, leading to unintended changes in the original data.
-
Overusing JSON Methods:
- While JSON.stringify/JSON.parse is simple, it doesn’t work for all objects (e.g., those containing methods or circular references).
-
Neglecting Performance:
- Deep copy methods can be slower, especially for large objects, so use them judiciously.
Conclusion
Understanding the difference between shallow copy and deep copy is essential for writing bug-free JavaScript code. Shallow copies are efficient for flat structures, while deep copies are indispensable for complex, nested objects. Choose the appropriate method based on your data structure and application needs, and avoid potential pitfalls by knowing the limitations of each approach.
? Download eBook - JavaScript: from ES2015 to ES2023
The above is the detailed content of JavaScript Shallow Copy vs Deep Copy: Examples and Best Practices. For more information, please follow other related articles on the PHP Chinese website!
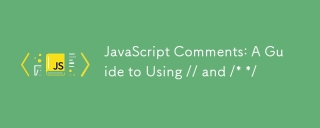
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
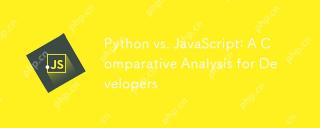
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
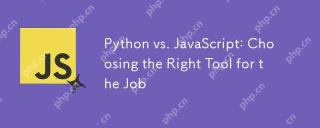
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
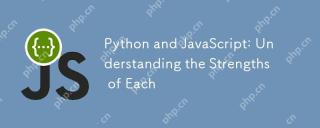
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
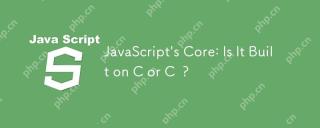
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
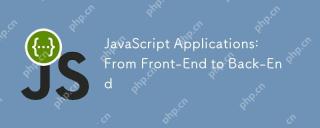
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
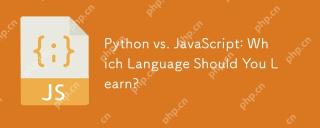
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
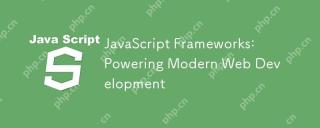
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
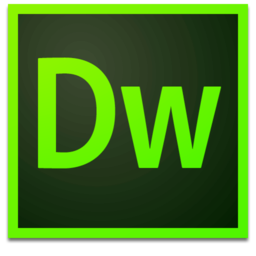
Dreamweaver Mac version
Visual web development tools
