


Advantage of sync.WaitGroup over Channels
In concurrent programming, synchronization between goroutines is crucial. While both sync.WaitGroup and channels provide means for synchronization, they differ in their approach and usage.
sync.WaitGroup
sync.WaitGroup, as the name suggests, provides a way to wait for a group of goroutines to complete their tasks. It operates by maintaining a count of active goroutines, and each goroutine signals its completion by calling the Done() method. The Wait() method blocks the calling goroutine until all goroutines in the group have called Done().
Example:
import ( "fmt" "sync" "time" ) var wg sync.WaitGroup func main() { words := []string{"foo", "bar", "baz"} for _, word := range words { wg.Add(1) go func(word string) { time.Sleep(1 * time.Second) defer wg.Done() fmt.Println(word) }(word) } // Waits for goroutines to finish wg.Wait() }
Advantages:
- Simplicity: Easier to understand and implement.
- Blocking: Blocks the calling goroutine until all goroutines complete, ensuring execution order.
- Scalability: Can handle multiple groups of goroutines with ease.
Channels
Channels, on the other hand, provide a way to communicate and exchange data between goroutines. They operate by sending and receiving values through channels, and multiple goroutines can concurrently read or write to the same channel.
Example:
import ( "fmt" "time" ) func main() { words := []string{"foo", "bar", "baz"} done := make(chan bool) for _, word := range words { go func(word string) { time.Sleep(1 * time.Second) fmt.Println(word) done <p><strong>Advantages:</strong></p>
- Communication: Allows goroutines to communicate and exchange data.
- Non-blocking: Does not block the calling goroutine, allowing for more concurrency.
- Flexibility: Can be used for more complex synchronization patterns.
Conclusion:
sync.WaitGroup is often preferred for simple synchronization tasks where blocking is desired. It provides a straightforward and scalable approach to ensure that all goroutines complete their tasks before proceeding. On the other hand, channels offer more flexibility and control over communication and synchronization, making them suitable for more complex scenarios.
The above is the detailed content of When should you use sync.WaitGroup over channels for synchronization in Go?. For more information, please follow other related articles on the PHP Chinese website!
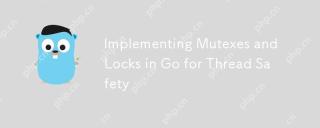
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
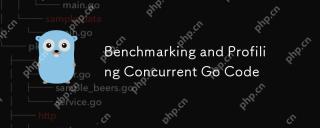
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
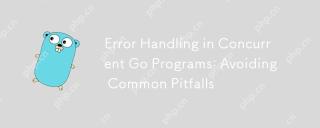
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.
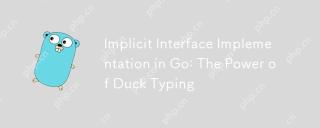
ImplicitinterfaceimplementationinGoembodiesducktypingbyallowingtypestosatisfyinterfaceswithoutexplicitdeclaration.1)Itpromotesflexibilityandmodularitybyfocusingonbehavior.2)Challengesincludeupdatingmethodsignaturesandtrackingimplementations.3)Toolsli
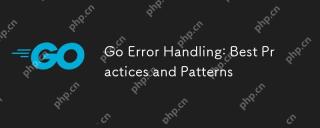
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
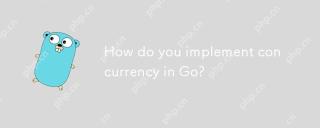
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
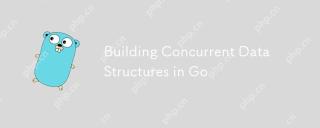
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
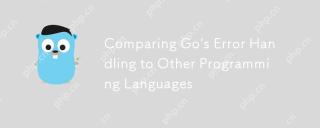
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
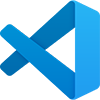
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
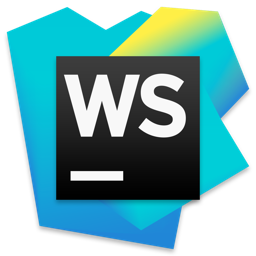
WebStorm Mac version
Useful JavaScript development tools
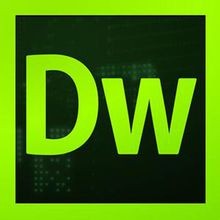
Dreamweaver CS6
Visual web development tools
