Obtaining Webpage Content as a String with Go
Introduction
In the world of web development, accessing the content of a webpage is often a crucial step for various tasks. Go, a versatile programming language, provides robust libraries for accomplishing this task.
Creating the OnPage Function
Your objective is to devise a function, OnPage, that takes a webpage's URL as a parameter and returns its content as a string. This string can then be processed and manipulated as desired.
Leveraging the HTTP Package
To establish a connection with a webpage and retrieve its content, Go's http package is indispensable. It provides the essential tools for sending HTTP requests and receiving responses.
Implementing OnPage Functionality
The following code snippet demonstrates how to implement the OnPage function:
package main import ( "fmt" "io/ioutil" "log" "net/http" ) func OnPage(link string) string { // Establish a connection with the webpage via HTTP GET res, err := http.Get(link) if err != nil { log.Fatal(err) } // Read the response body, which contains the webpage's content content, err := io.ReadAll(res.Body) if err != nil { log.Fatal(err) } // Close the response body res.Body.Close() // Convert the content into a string and return it return string(content) } func main() { // Example usage: retrieve the content of the BBC News UK webpage fmt.Println(OnPage("http://www.bbc.co.uk/news/uk-england-38003934")) }
This enhanced implementation constructs an HTTP request, sends it to the specified URL, and retrieves the response body containing the webpage's content. It then converts the response content into a string and returns it. The main function demonstrates the function's usage by fetching the content of a webpage and printing it to the console.
The above is the detailed content of How Can I Retrieve Webpage Content as a String Using Go?. For more information, please follow other related articles on the PHP Chinese website!
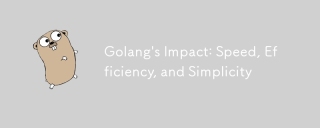
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
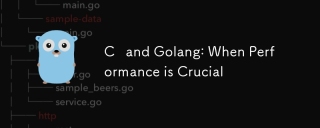
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
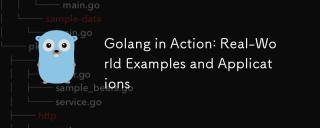
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
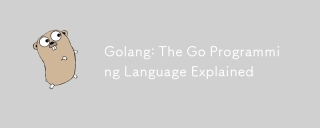
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
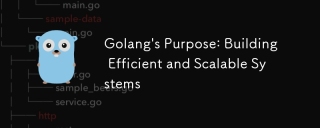
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
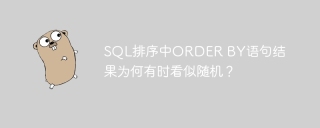
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
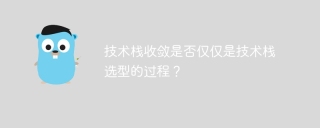
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
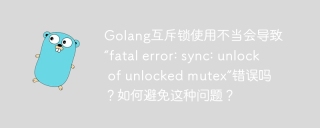
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
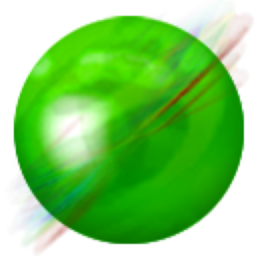
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
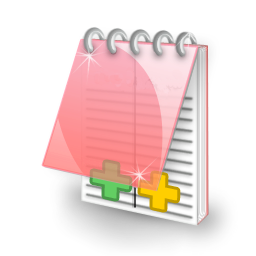
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
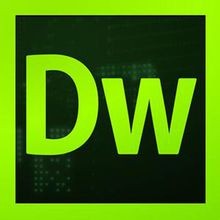
Dreamweaver CS6
Visual web development tools