


Preserving Existing File Content: Appending to a File in Node
Appending data to a file in Node while maintaining existing content can be tricky, as demonstrated by the writeFile method's behavior. To overcome this challenge, consider utilizing the appendFile method:
1. Asynchronous Appends with appendFile
const fs = require('fs'); fs.appendFile('message.txt', 'data to append', function (err) { if (err) throw err; console.log('Saved!'); });
2. Synchronous Appends with appendFileSync
const fs = require('fs'); fs.appendFileSync('message.txt', 'data to append');
These methods perform asynchronous or synchronous appends, respectively, using a new file handle each time they're invoked.
3. File Handle Reuse
However, for frequent appends to the same file, it's recommended to reuse the file handle to enhance efficiency. This can be achieved using the fs.open method:
const fs = require('fs'); fs.open('message.txt', 'a', function(err, fd) { if (err) throw err; // Append data using the file handle fs.write(fd, 'data to append', function(err) { if (err) throw err; }); // Close the file handle when finished fs.close(fd, function(err) { if (err) throw err; }); });
The above is the detailed content of How Can I Append Data to a File in Node.js While Preserving Existing Content?. For more information, please follow other related articles on the PHP Chinese website!
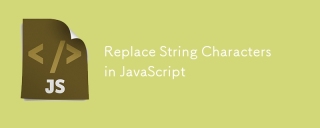
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
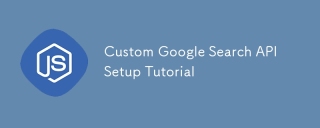
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
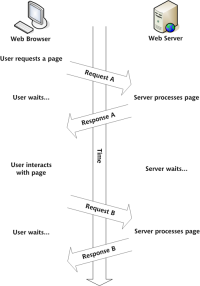
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
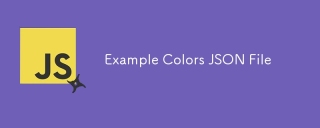
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
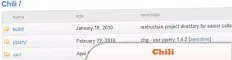
Enhance Your Code Presentation: 10 Syntax Highlighters for Developers Sharing code snippets on your website or blog is a common practice for developers. Choosing the right syntax highlighter can significantly improve readability and visual appeal. T
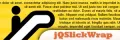
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
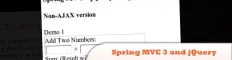
This article presents a curated selection of over 10 tutorials on JavaScript and jQuery Model-View-Controller (MVC) frameworks, perfect for boosting your web development skills in the new year. These tutorials cover a range of topics, from foundatio
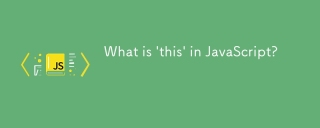
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
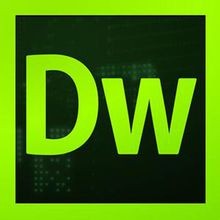
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
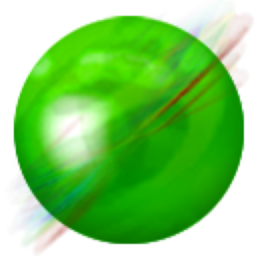
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
