


How to Optimize Parameter Passing in C : Lvalues, Rvalues, and Best Practices?
How to Pass Parameters Effectively in C
In C , there are specific guidelines for passing parameters correctly to optimize efficiency while maintaining clarity in your code.
Passing Modes
Pass by lvalue reference: Use this when the function needs to modify the original object passed, with changes being visible to the caller.
Pass by lvalue reference to const: Choose this when the function needs to observe the object's state without modifying it or creating a copy.
Pass by value: Opt for this when the function doesn't modify the original object and only needs to observe it. It's preferred for fundamental types where copying is fast.
Handling Rvalues
For rvalues, pass by rvalue reference: This avoids unnecessary moves or copies. Use perfect forwarding to handle both lvalues and rvalues, ensuring efficient binding.
Handling Expensive Moves
Use constructor overloads: Define overloads for lvalue references and rvalue references. This allows the compiler to select the correct overload based on the parameter type, ensuring no unnecessary copies or moves.
Example Application
Let's revisit the CreditCard example considering these guidelines:
Pass CreditCard by rvalue reference:
Account(std::string number, float amount, CreditCard&& creditCard): number(number) , amount(amount) , creditCard(std::forward<creditcard>(creditCard)) {}</creditcard>
This ensures a move from the rvalue CreditCard passed as an argument.
Use constructor overloads:
Account(std::string number, float amount, const CreditCard& creditCard): number(number) , amount(amount) , creditCard(creditCard) {} Account(std::string number, float amount, CreditCard&& creditCard): number(number) , amount(amount) , creditCard(std::move(creditCard)) {}
This allows the compiler to select the correct overload, either copying from an lvalue or moving from an rvalue.
By applying these guidelines, you can optimize parameter passing in C , maintaining code clarity and efficiency.
The above is the detailed content of How to Optimize Parameter Passing in C : Lvalues, Rvalues, and Best Practices?. For more information, please follow other related articles on the PHP Chinese website!
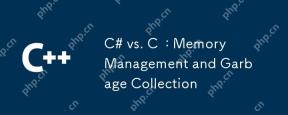
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
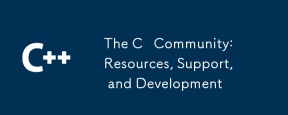
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
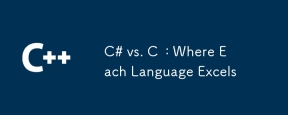
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
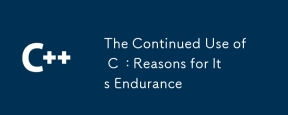
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
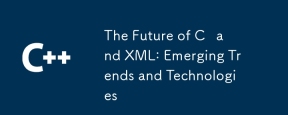
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
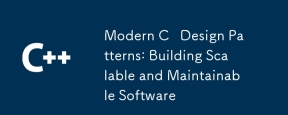
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
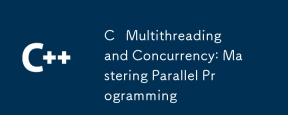
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
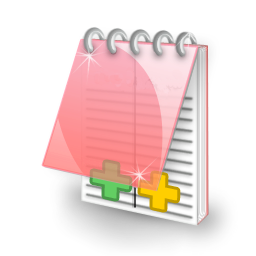
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
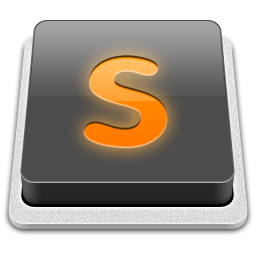
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.