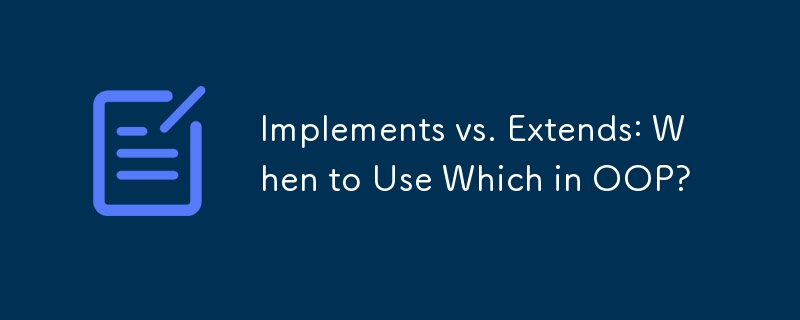
When to Use "Implements" vs "Extends" in Object-Oriented Programming
In object-oriented programming, understanding the difference between "implements" and "extends" is crucial. While both keywords allow classes to inherit functionality, they have distinct purposes.
Implements Interface vs Extends Class
-
Implements Interface: Used when a class agrees to provide implementations for methods declared in an interface. Interfaces define contracts that classes must fulfill by implementing the specified methods.
-
Extends Class: Used when a class inherits the properties and methods of a parent class. It allows the subclass to reuse and extend the functionality of the parent class.
Key Differences
-
Inheritance: Extends establishes a parent-child relationship, allowing the subclass to access the methods and variables defined in the parent class. Implements, on the other hand, does not provide direct inheritance.
-
Multiple Implementation: Interfaces support multiple inheritance in Java, allowing a class to implement multiple interfaces. Classes, however, only support single inheritance.
-
Overriding vs Implementing: When implementing an interface, the class must provide implementations for all declared methods. When extending a class, the subclass can optionally override parent class methods to modify their behavior.
When to Use
-
Implements: Use when you want a class to fulfill a specific contract defined by an interface, particularly if multiple inheritance is required.
-
Extends: Use when you want to extend the functionality of an existing class and reuse its methods and variables.
Examples
-
Implements:
public interface Drawable {
void draw();
}
public class Circle implements Drawable {
@Override
public void draw() {
// Implement the circle drawing logic
}
}
-
Extends:
public class Base {
private int value;
public int getValue() {
return value;
}
}
public class Child extends Base {
private String name;
public String getName() {
return name;
}
}
Understanding the nuances between "implements" and "extends" is essential for designing effective and maintainable Java code.
The above is the detailed content of Implements vs. Extends: When to Use Which in OOP?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn