Time complexity is considered one of the toughest topics for beginners who are just starting with Problem-Solving. Here, I am providing the time complexity analysis cheat sheet. I hope this helps. Please let me know if you have any questions.
Time Complexity Analysis Cheatsheet
Quick Reference Table
O(1) - Constant time O(log n) - Logarithmic (halving/doubling) O(n) - Linear (single loop) O(n log n) - Linearithmic (efficient sorting) O(n²) - Quadratic (nested loops) O(2ⁿ) - Exponential (recursive doubling) O(n!) - Factorial (permutations)
Identifying Patterns
1. O(1) - Constant
# Look for: - Direct array access - Basic math operations - Fixed loops - Hash table lookups # Examples: arr[0] x + y for i in range(5) hashmap[key]
2. O(log n) - Logarithmic
# Look for: - Halving/Doubling - Binary search patterns - Tree traversal by level # Examples: while n > 0: n = n // 2 left, right = 0, len(arr)-1 while left <h3> 3. O(n) - Linear </h3> <pre class="brush:php;toolbar:false"># Look for: - Single loops - Array traversal - Linear search - Hash table building # Examples: for num in nums: # O(1) operation total += num for i in range(n): # O(1) operation arr[i] = i
4. O(n log n) - Linearithmic
# Look for: - Efficient sorting - Divide and conquer - Tree operations with traversal # Examples: nums.sort() sorted(nums) merge_sort(nums)
5. O(n²) - Quadratic
# Look for: - Nested loops - Simple sorting - Matrix traversal - Comparing all pairs # Examples: for i in range(n): for j in range(n): # O(1) operation # Pattern finding for i in range(n): for j in range(i+1, n): # Compare pairs
6. O(2ⁿ) - Exponential
# Look for: - Double recursion - Power set - Fibonacci recursive - All subsets # Examples: def fib(n): if n <h2> Common Operations Time Complexity </h2> <h3> Array/List Operations </h3> <pre class="brush:php;toolbar:false"># O(1) arr[i] # Access arr.append(x) # Add end arr.pop() # Remove end # O(n) arr.insert(i, x) # Insert middle arr.remove(x) # Remove by value arr.index(x) # Find index min(arr), max(arr) # Find min/max
Dictionary/Set Operations
# O(1) average d[key] # Access d[key] = value # Insert key in d # Check existence d.get(key) # Get value # O(n) len(d) # Size d.keys() # Get keys d.values() # Get values
String Operations
# O(n) s + t # Concatenation s.find(t) # Substring search s.replace(old, new) # Replace ''.join(list) # Join # O(n²) potential s += char # Repeated concatenation
Loop Analysis
Single Loop
# O(n) for i in range(n): # O(1) operations # O(n/2) = O(n) for i in range(0, n, 2): # Skip elements still O(n)
Nested Loops
# O(n²) for i in range(n): for j in range(n): # O(1) operations # O(n * m) for i in range(n): for j in range(m): # Different sizes # O(n²/2) = O(n²) for i in range(n): for j in range(i, n): # Triangular still O(n²)
Multiple Loops
# O(n + m) for i in range(n): # O(1) for j in range(m): # O(1) # O(n + n²) = O(n²) for i in range(n): # O(1) for i in range(n): for j in range(n): # O(1)
Recursive Analysis
Linear Recursion
# O(n) def factorial(n): if n <h3> Binary Recursion </h3> <pre class="brush:php;toolbar:false"># O(2ⁿ) def fibonacci(n): if n <h3> Divide & Conquer </h3> <pre class="brush:php;toolbar:false"># O(n log n) def mergeSort(arr): if len(arr) <h2> Optimization Red Flags </h2> <h3> Hidden Loops </h3> <pre class="brush:php;toolbar:false"># String operations for c in string: newStr += c # O(n²) # List comprehension [x for x in range(n) for y in range(n)] # O(n²)
Built-in Functions
len() # O(1) min(), max() # O(n) sorted() # O(n log n) list.index() # O(n)
Tips for Analysis
- Count nested loops
- Check recursive branching
- Consider hidden operations
- Look for divide & conquer
- Check built-in function complexity
- Consider average vs worst case
- Watch for loop variables
- Consider input constraints
Thank you for reading, please give the thumbs up on the post if you found this helpful. Cheers!
The above is the detailed content of Easy way to find the Time Complexity of an Algorithm. For more information, please follow other related articles on the PHP Chinese website!
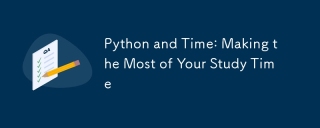
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
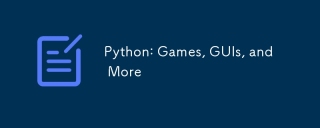
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
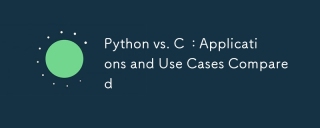
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
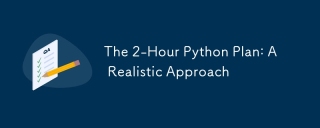
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
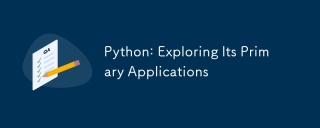
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
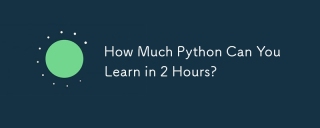
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
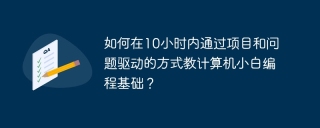
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
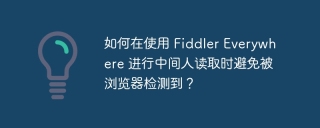
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
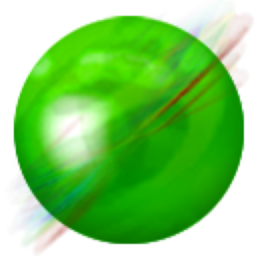
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use
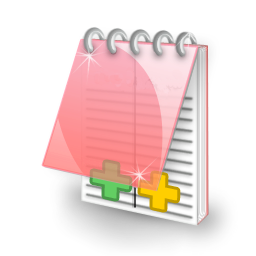
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function