


Why Does Pointer Decay Override Template Deduction in C Function Overloading?
Pointer Decay Takes Precedence Over Template Deduction
When writing code that operates on strings, it is common to encounter a dilemma where attempting to overload a function to accommodate both array-based and non-array-based string representations can result in unexpected behavior.
In this specific scenario, an initial function foo is defined to print the length of an array of characters:
template <size_t n> void foo(const char (&s)[N]) { std::cout <p>This function accepts arrays of characters and outputs the array length. However, when extending <strong>foo</strong> to also handle non-array strings, ambiguity arises:</p> <pre class="brush:php;toolbar:false">void foo(const char* s) { std::cout <p>The intention is for the second <strong>foo</strong> overload to handle non-array strings, but surprisingly, this extension leads to the original overload being bypassed for both array and non-array strings:</p><pre class="brush:php;toolbar:false">foo("hello") // prints raw, size=5
This unexpected behavior stems from the concept of pointer decay. Arrays are essentially pointers to their first element, and converting an array to a pointer is a very inexpensive operation. As a result, the compiler prioritizes the overload that accepts a pointer parameter, even though it requires an implicit conversion from the array argument.
To ensure the desired behavior, where the array-based overload is used for arrays, it is necessary to introduce an additional overload:
template <typename t> auto foo(T s) -> std::enable_if_t<:is_convertible char const>{}> { std::cout <p>This overload uses template deduction and restricts its applicability to types that can be converted to character pointers. Through partial ordering, the compiler now correctly selects the appropriate <strong>foo</strong> function based on the argument type.</p></:is_convertible></typename>
The above is the detailed content of Why Does Pointer Decay Override Template Deduction in C Function Overloading?. For more information, please follow other related articles on the PHP Chinese website!
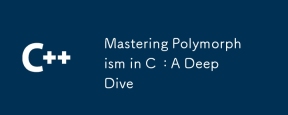
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
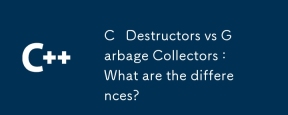
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
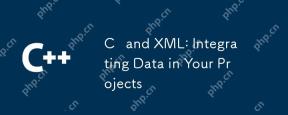
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
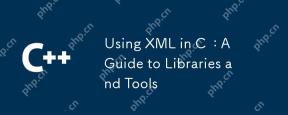
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
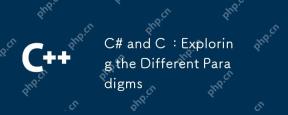
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
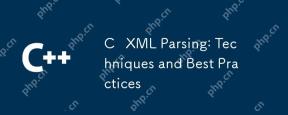
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
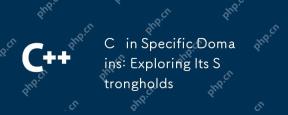
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
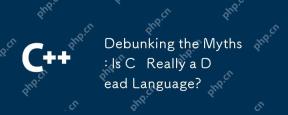
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
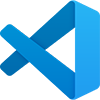
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
