Key Order in Maps: A Mysterious Anomaly Explained
In Go, maps are powerful structures that allow for efficient data organization and retrieval. However, map ordering can be a source of confusion, as evidenced in the code snippet below:
package main import "fmt" type Vertex struct { Lat, Long float64 } var m map[string]Vertex func main() { m = make(map[string]Vertex) m["Bell Labs"] = Vertex{ 40.68433, 74.39967, } m["test"] = Vertex{ // Move the right "}" 4 spaces 12.0, 100, } // Missing closing bracket fmt.Println(m["Bell Labs"]) fmt.Println(m) }
When we run this code, we observe a peculiar behavior:
{40.68433 74.39967} map[test:{12 100} Bell Labs:{40.68433 74.39967}]
Why does this subtle modification in the test vertex declaration alter the order of the map?
Understanding Map Order
Contrary to popular belief, maps in Go are not ordered in the sense that elements are arranged in a specific sequence. Instead, they are implemented using a hash table, where each key is mapped to a unique hash value. This hash value is then used to determine the location of the corresponding element in the table.
Impact of Hash Functions
The order of keys in the map is largely determined by the hash function used. Hash functions are algorithms that convert an input value, such as a string or an object, into a numeric value. In Go, the hash function is randomized to make it difficult to predict the hash value associated with a particular key.
This randomization is a crucial security feature designed to prevent denial-of-service attacks. It ensures that attackers cannot generate hash collisions, where multiple keys have the same hash value, to manipulate data in the hash table.
Documentation and Implementation Considerations
It's important to note that the Go specification explicitly states that map order is not guaranteed. This means that the order may vary between different implementations or even for the same implementation across different execution environments.
While current Go implementations maintain the order of inserted keys, it is not wise to rely on this behavior. Future implementations could introduce optimizations or rearrange elements for performance or stability reasons.
The above is the detailed content of Why Is the Order of Keys in Go Maps Seemingly Unpredictable?. For more information, please follow other related articles on the PHP Chinese website!
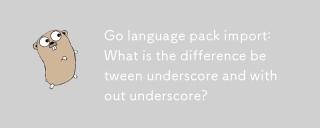
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
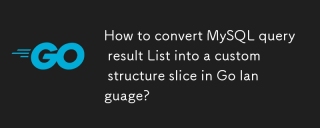
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
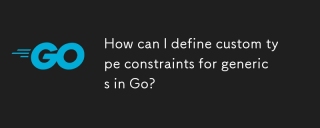
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
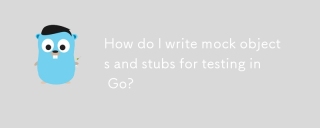
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
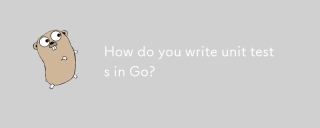
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
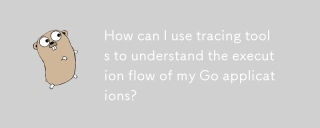
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
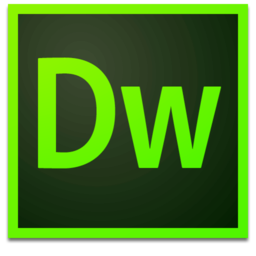
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
