


How Can JUnit 5\'s `assertThrows()` Optimize Multiple Exception Verification in Tests?
JUnit 5 Exception Assertions: Optimizing for Multiple Exception Verification
In JUnit 5, testing for exceptions has been simplified with the introduction of assertThrows(). This approach provides a convenient and concise way to verify that methods throw expected exceptions, addressing the limitations of using @Rule for handling multiple exceptions within a test.
Improved Exception Testing with assertThrows()
assertThrows() allows you to define an assertion for a specific exception type and execute a lambda expression that triggers the exception. By incorporating this approach, you can evaluate multiple exceptions within a single test. Here's an example:
import static org.junit.jupiter.api.Assertions.assertThrows; @Test void exceptionTesting() { MyException thrown = assertThrows( MyException.class, () -> myObject.doThing(), "Expected doThing() to throw, but it didn't" ); assertTrue(thrown.getMessage().contains("Stuff")); }
The above is the detailed content of How Can JUnit 5\'s `assertThrows()` Optimize Multiple Exception Verification in Tests?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
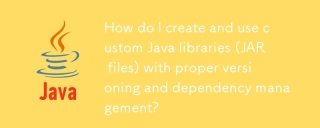
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
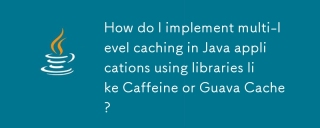
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
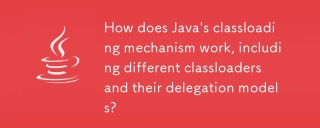
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
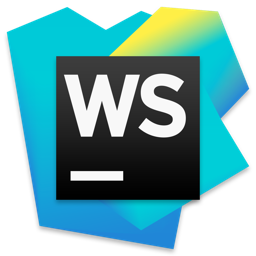
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
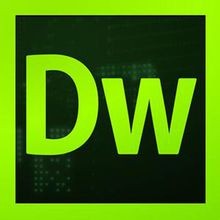
Dreamweaver CS6
Visual web development tools