


Understanding Prototype Sharing in JavaScript: Objects Inherited from Prototypes
In JavaScript, objects inherit properties from their prototypes. One aspect to consider is how arrays defined as prototype properties behave within class instances.
As observed, prototyped arrays are not private to individual class instances but are instead shared among all instances. This behavior arises because the prototype of an object acts as an object itself. Prototype properties are shared by all objects derived from that specific prototype.
Consider the following example:
function Sandwich() { // Uncomment this to fix the problem //this.ingredients = []; } Sandwich.prototype = { "ingredients" : [], "addIngredients" : function( ingArray ) { for( var key in ingArray ) { this.addIngredient( ingArray[ key ] ); } }, "addIngredient" : function( thing ) { this.ingredients.push( thing ); } }
In this example, an array named "ingredients" is defined as a prototype property of the "Sandwich" function. If "this.ingredients = [];" is commented out within the constructor, the following behavior occurs:
When a new instance of "Sandwich" is created (such as "cheeseburger" or "blt"), these instances initially inherit the "ingredients" array from the prototype. Modifications made to this array, such as adding ingredients to "cheeseburger," also affect the "ingredients" arrays of all other "Sandwich" instances. This shared behavior arises from the fact that all instances reference the same array, not individual copies.
To resolve this issue and create a separate array for each instance, the "ingredients" array must be defined within the constructor:
function Sandwich() { this.ingredients = []; }
This modification assigns a new array to each instance of "Sandwich" during construction, ensuring that any changes made to the "ingredients" array are instance-specific.
In summary, arrays defined as prototype properties are shared among all objects that inherit from that prototype. To create instance-specific data, such as arrays, they must be defined within the constructor. This understanding is crucial for effective use of inheritance and object-oriented programming in JavaScript.
The above is the detailed content of Why Do Prototype Arrays in JavaScript Get Shared Across Class Instances?. For more information, please follow other related articles on the PHP Chinese website!
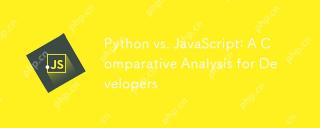
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
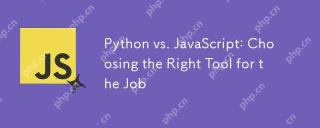
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
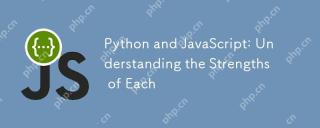
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
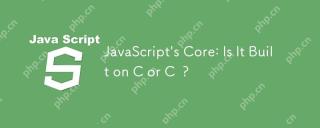
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
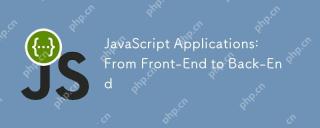
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
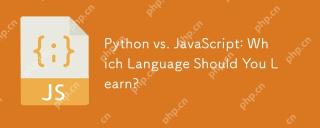
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
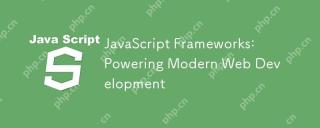
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
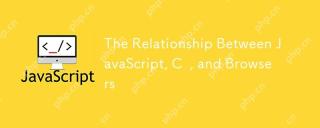
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
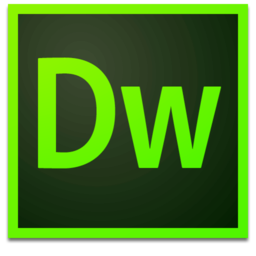
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
