How to Convert Byte Size into Human-Readable Format in Java
Converting byte size into a human-readable format is a common task in software development. In Java, there's no built-in method for this, but we can use Apache Commons Lang3 for a handy way to do it.
Apache Commons Lang3 provides two methods for humanizing byte counts:
- humanReadableByteCountSI: Uses the International System of Units (SI), where 1024 bytes is 1 kilobyte (similar to how 1000 grams is 1 kilogram).
- humanReadableByteCountBin: Uses a binary system, where 1024 bytes is 1 kibibyte (similar to how 1024 bits is 1 kilobit).
Here's how to use these methods:
import org.apache.commons.lang3.StringUtils; public class ByteSizeConverter { public static void main(String[] args) { long bytes = 1024 * 1024; // Convert to SI format (e.g., 1 MB) String humanReadableBytesSI = StringUtils.humanReadableByteCountSI(bytes); System.out.println(humanReadableBytesSI); // "1 MB" // Convert to binary format (e.g., 1 MiB) String humanReadableBytesBin = StringUtils.humanReadableByteCountBin(bytes); System.out.println(humanReadableBytesBin); // "1 MiB" } }
These methods provide a convenient way to represent byte sizes in a human-readable format, regardless of whether you prefer SI or binary units.
The above is the detailed content of How Can I Convert Bytes to a Human-Readable Format in Java?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
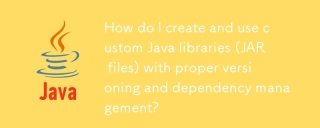
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
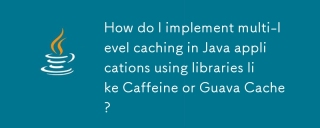
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
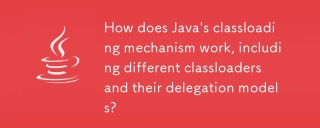
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
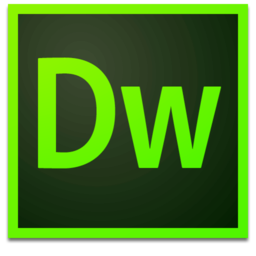
Dreamweaver Mac version
Visual web development tools