


Inserting a Value into a Slice at a Given Index
Question:
How can we insert a value into a specific index in a Go slice without including other elements?
Problem Description:
Suppose we have two slices, array1 and array2, and we want to insert array2[2] at array1[1]. We want to keep the rest of array1 untouched.
Background:
Earlier techniques involved using the colon operator (:), but it also includes subsequent elements. This tutorial aims to provide a comprehensive solution focused on inserting single values at a specific index.
Solution:
Using the slices.Insert Package (Go 1.21 ):
result := slices.Insert(slice, index, value)
Note: 0 ≤ index ≤ len(slice)
Using Append and Assignment
a = append(a[:index+1], a[index:]...) a[index] = value
Note: len(a) > 0 && index
For special cases:
-
If len(a) == index, do:
a = append(a, value)
-
If inserting at index zero and dealing with an int slice, do:
a = append([]int{value}, a...)
Custom Function:
func insert(a []int, index int, value int) []int { if len(a) == index { return append(a, value) } a = append(a[:index+1], a[index:]...) a[index] = value return a }
Generic Function:
func insert[T any](a []T, index int, value T) []T { ... return a }
Example:
slice1 := []int{1, 3, 4, 5} slice2 := []int{2, 4, 6, 8} slice1 = append(slice1[:2], slice1[1:]...) slice1[1] = slice2[2] fmt.Println(slice1) // [1 6 3 4 5]
The above is the detailed content of How to Insert a Value into a Go Slice at a Specific Index Without Affecting Other Elements?. For more information, please follow other related articles on the PHP Chinese website!
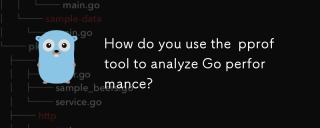
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
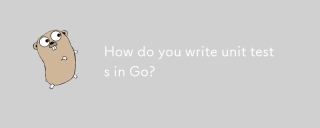
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
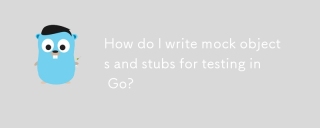
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
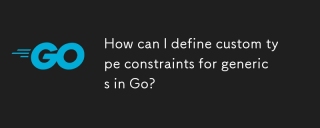
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
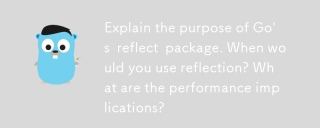
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
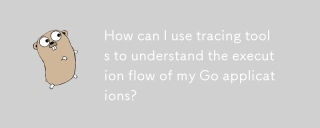
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
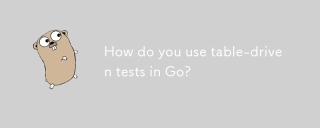
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
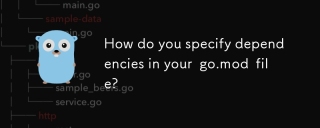
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
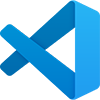
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
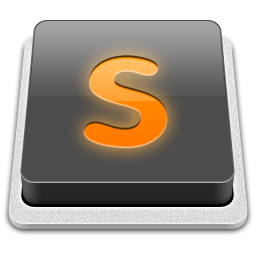
SublimeText3 Mac version
God-level code editing software (SublimeText3)
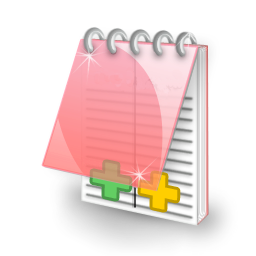
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
