Removing Leading and Trailing Spaces from a String in C
String manipulation in C often involves removing unnecessary spaces from strings. This can be particularly useful for tasks such as data cleaning or text processing.
Removing Leading and Trailing Spaces
To remove leading and trailing spaces, one can employ the find_first_not_of and find_last_not_of methods. These functions find the first and last non-whitespace characters in a string, respectively. Using these values, one can obtain the substring that contains the non-whitespace characters:
std::string trim(const std::string& str, const std::string& whitespace = " \t") { const auto strBegin = str.find_first_not_of(whitespace); if (strBegin == std::string::npos) return ""; // no content const auto strEnd = str.find_last_not_of(whitespace); const auto strRange = strEnd - strBegin + 1; return str.substr(strBegin, strRange); }
Removing Extra Spaces Between Words
To remove extra spaces between words, an additional step is required. This can be achieved using the find_first_of, find_last_of, find_first_not_of, and find_last_not_of methods along with substr to replace the extra spaces with a single space:
std::string reduce(const std::string& str, const std::string& fill = " ", const std::string& whitespace = " \t") { // trim first auto result = trim(str, whitespace); // replace sub ranges auto beginSpace = result.find_first_of(whitespace); while (beginSpace != std::string::npos) { const auto endSpace = result.find_first_not_of(whitespace, beginSpace); const auto range = endSpace - beginSpace; result.replace(beginSpace, range, fill); const auto newStart = beginSpace + fill.length(); beginSpace = result.find_first_of(whitespace, newStart); } return result; }
Example Usage
The following code snippet demonstrates the usage of these functions:
const std::string foo = " too much\t \tspace\t\t\t "; const std::string bar = "one\ntwo"; std::cout <p>Output:</p><pre class="brush:php;toolbar:false">[too much space] [too much space] [too-much-space] [one two]
The above is the detailed content of How to Remove Leading, Trailing, and Extra Spaces from a String in C ?. For more information, please follow other related articles on the PHP Chinese website!
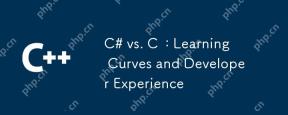
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
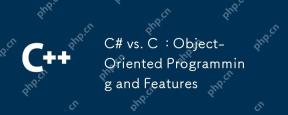
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
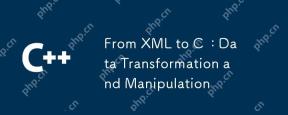
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
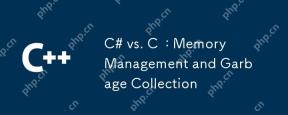
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
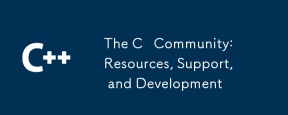
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
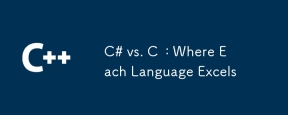
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
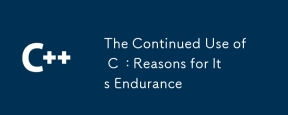
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
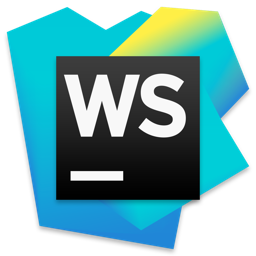
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor