Creating a Thread Pool with Boost in C
When working with computationally intensive tasks, it can be beneficial to utilize a thread pool to efficiently manage and distribute these tasks across multiple threads. Boost provides a powerful toolset for creating thread pools in C .
Creating the Thread Pool
To create a thread pool using Boost, start by creating an asio::io_service instance (ioService) and a thread_group instance (threadpool). The thread_group will contain the worker threads that will execute the tasks.
boost::asio::io_service ioService; boost::thread_group threadpool;
Filling the Thread Pool
To fill the thread pool with threads, use the create_thread member function of the thread_group to create threads and link them to the ioService.
threadpool.create_thread( boost::bind(&boost::asio::io_service::run, &ioService) );
Assigning Tasks to the Thread Pool
Tasks can be assigned to the thread pool using the post member function of the ioService. Pass a boost::bind object as an argument to the post function. The boost::bind object encapsulates the function to be executed and any arguments it requires.
ioService.post(boost::bind(myTask, "Hello World!"));
Stopping the Thread Pool
Once all tasks have been assigned and completed, the thread pool can be stopped. Call the stop member function of the ioService to stop the processing loop.
ioService.stop();
Finally, join the threads in the thread pool using the join_all member function of the thread_group to ensure all threads have finished executing before proceeding.
threadpool.join_all();
By following these steps, you can create and manage a thread pool in C using Boost. This approach provides a flexible and efficient way to handle parallel tasks, improving the performance of your application.
The above is the detailed content of How can I efficiently create and manage a thread pool in C using Boost?. For more information, please follow other related articles on the PHP Chinese website!
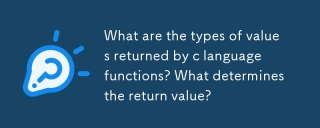
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
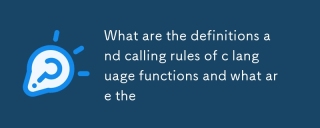
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
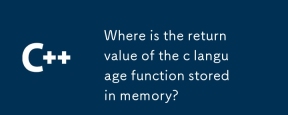
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
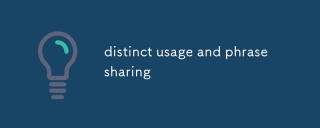
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
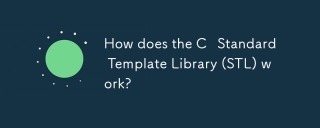
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
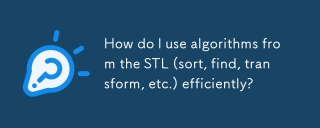
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version
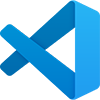
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
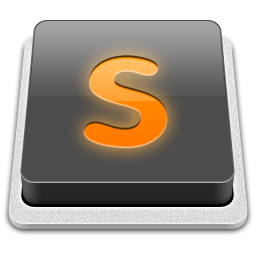
SublimeText3 Mac version
God-level code editing software (SublimeText3)
