


How do you add a header and footer to a RecyclerView without getting a NullPointerException?
Implementing Headers and Footers in RecyclerView
Problem:
Adding a header or footer to a RecyclerView can be a challenge. The code provided suggests adding a view to the LinearLayoutManager using addView(View view, int position), but this approach was not successful and resulted in a NullPointerException.
Solution:
Adding a Footer
To add a footer to a RecyclerView, follow these steps:
- Create a ViewHolder for the footer view.
- Override onCreateViewHolder to inflate the footer view when necessary.
- Override onBindViewHolder to bind data to the footer view.
- Override getItemCount to include the footer in the count.
- Override getItemViewType to distinguish the footer from the normal list items.
Example Footer Adapter:
public class FooterViewHolder extends RecyclerView.ViewHolder { public FooterViewHolder(View itemView) { super(itemView); } } public class RecyclerViewWithFooterAdapter extends RecyclerView.Adapter<recyclerview.viewholder> { private static final int FOOTER_VIEW = 1; private List<string> data; @Override public int getItemCount() { return data == null ? 0 : data.size() + 1; } @Override public int getItemViewType(int position) { return position == data.size() ? FOOTER_VIEW : super.getItemViewType(position); } @Override public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { View v; if (viewType == FOOTER_VIEW) { v = LayoutInflater.from(context).inflate(R.layout.list_item_footer, parent, false); return new FooterViewHolder(v); } v = LayoutInflater.from(context).inflate(R.layout.list_item_normal, parent, false); return new NormalViewHolder(v); } @Override public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) { if (holder instanceof FooterViewHolder) { FooterViewHolder vh = (FooterViewHolder) holder; } else { NormalViewHolder vh = (NormalViewHolder) holder; vh.bindView(position); } } }</string></recyclerview.viewholder>
Adding a Header
Adding a header to a RecyclerView follows a similar process:
- Create a ViewHolder for the header view.
- Override onCreateViewHolder to inflate the header view when necessary.
- Add the header view to the RecyclerView using RecyclerView.addHeaderView(View view).
- Override getItemCount to include the header in the count.
Additional Notes:
- If you need multiple headers and footers, handle them similarly by adjusting the getItemViewType and getItemCount methods.
- The provided GitHub repository offers a complete implementation of adding both headers and footers.
The above is the detailed content of How do you add a header and footer to a RecyclerView without getting a NullPointerException?. For more information, please follow other related articles on the PHP Chinese website!
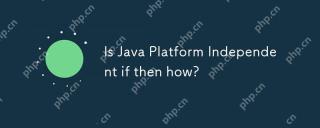
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
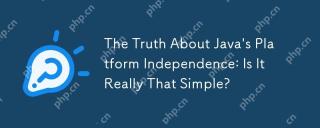
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
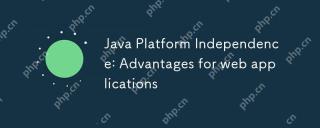
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
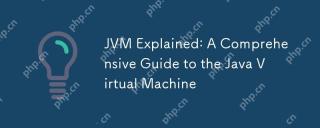
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
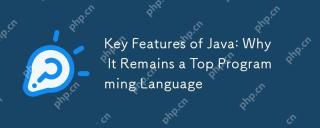
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
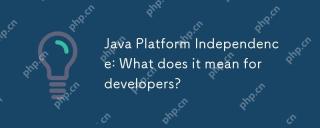
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
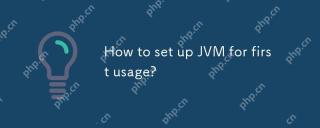
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
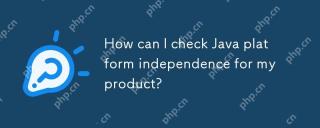
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
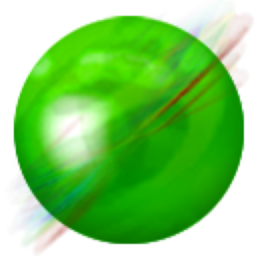
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
