


Exploring Techniques for Class Definition in JavaScript and Their Implications
In JavaScript, the need to define classes for implementing object-oriented programming is often encountered, especially in large-scale projects. While there is no explicit class keyword in JavaScript, several techniques exist to simulate class behavior. Let's delve into these techniques and explore their nuances.
Constructor Function Approach
The constructor function approach mimics class definition in other languages. Here's the syntax:
function Person(name, gender) { this.name = name; this.gender = gender; this.speak = function() { alert("Howdy, my name is" + this.name); }; }
In this approach, the Person function acts as the constructor, creating instances with the properties and methods defined within it.
Factory Function Approach
The factory function approach uses closures to create objects and is similar to the constructor function approach. Here's the syntax:
function createPerson(name, gender) { return { name: name, gender: gender, speak: function() { alert("Howdy, my name is" + this.name); } }; }
In this approach, the createPerson function returns a new object initialized with the given properties and methods.
Prototype-Based Inheritance
JavaScript implements a unique form of inheritance called prototype-based inheritance. Objects inherit properties and methods from their prototypes. Here's the syntax:
// Define a Person prototype var Person = { speak: function() { alert("Howdy, my name is" + this.name); } }; // Create a new object using the Person prototype var person = Object.create(Person); person.name = "Bob";
In this approach, the Person object serves as the prototype, providing a shared repository of properties and methods. New objects are created by creating a new object that inherits from the prototype.
Comparison and Trade-offs
Constructor Function:
- Pros: Familiar syntax, straightforward implementation
- Cons: No implicit prototype chain, verbose (multiple lines required to create an instance)
Factory Function:
- Pros: Encapsulated object creation, promotes code reuse
- Cons: Less familiar syntax, introduces extra function calls
Prototype-Based Inheritance:
- Pros: More flexible, provides implicit inheritance, reduces code duplication
- Cons: Can be difficult to understand for beginners, potential for prototype pollution
Conclusion
The choice of technique for defining classes in JavaScript depends on your specific project requirements. Consider the trade-offs discussed above and select the approach that best suits your needs. While the constructor function approach is the simplest, prototype-based inheritance provides a more elegant and flexible inheritance mechanism that is highly suitable for large-scale object-oriented JavaScript projects.
The above is the detailed content of How do you define classes in JavaScript, and what are the different approaches and their trade-offs?. For more information, please follow other related articles on the PHP Chinese website!

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
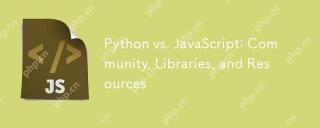
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
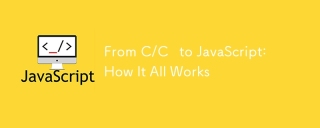
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
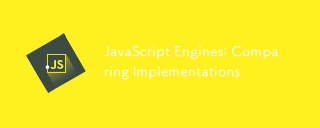
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
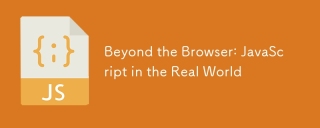
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
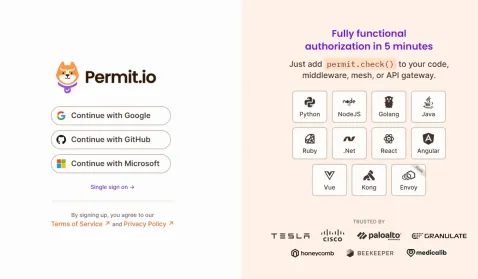
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
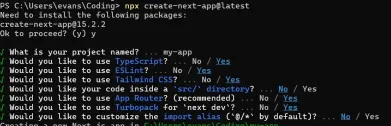
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
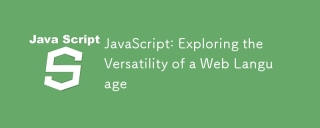
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
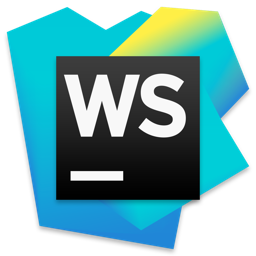
WebStorm Mac version
Useful JavaScript development tools
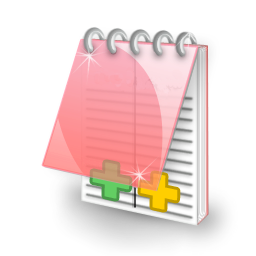
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
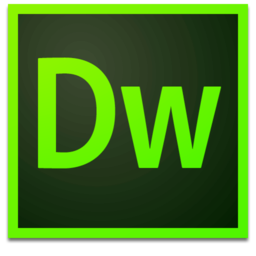
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.