Autocomplete Functionality in Java Using JTextfield and JList
In the realm of user interfaces, it is often desirable to provide a feature that allows users to easily enter data by suggesting potential completions based on the characters they type. In Java, this functionality can be achieved by utilizing the JTextfield component for user input and the JList component to display the suggestions.
The implementation of this autocomplete functionality involves two primary steps:
- Retrieve Data: Populate the JList with a list of potential completion options. This could be data obtained from a database, a local file, or an array.
- Implement Autocomplete Logic: Listen to input events from the JTextfield and dynamically filter the JList based on the user's input. This can be accomplished using methods to retrieve matching options from the data source or by utilizing Java's autocompletion algorithms.
To illustrate this concept further, we delve into a concise code example:
import java.util.ArrayList; import javax.swing.*; public class AutoComplete { private JFrame frame; private ArrayList<string> suggestions; private JTextfield textField; private JList<string> suggestionList; public AutoComplete() { // Populate suggestions suggestions = new ArrayList(); suggestions.add("Apple"); suggestions.add("Banana"); suggestions.add("Orange"); // Initialize components textField = new JTextfield(); suggestionList = new JList(); // Implement autocomplete logic textField.addKeyListener(new KeyListener() { @Override public void keyTyped(KeyEvent e) { // Filter suggestions based on user input String input = textField.getText(); DefaultListModel<string> listModel = new DefaultListModel(); for (String suggestion : suggestions) { if (suggestion.startsWith(input)) { listModel.addElement(suggestion); } } // Update the suggestion list suggestionList.setModel(listModel); } @Override public void keyPressed(KeyEvent e) {} @Override public void keyReleased(KeyEvent e) {} }); // Display the components frame = new JFrame(); frame.setLayout(new GridLayout(2, 1)); frame.add(textField); frame.add(suggestionList); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.pack(); frame.setVisible(true); } public static void main(String[] args) { SwingUtilities.invokeLater(new Runnable() { @Override public void run() { new AutoComplete(); } }); } }</string></string></string>
In this example, user input is filtered against a prepopulated list of suggestions. As the user types, the suggestion list is dynamically updated to display only matching options. By utilizing this approach, the developer can provide users with a faster and more efficient data entry process.
The above is the detailed content of How to Implement Autocomplete Functionality in Java using JTextField and JList?. For more information, please follow other related articles on the PHP Chinese website!
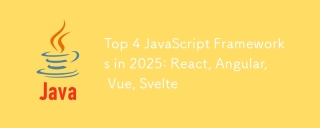
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
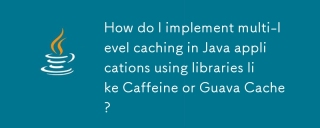
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
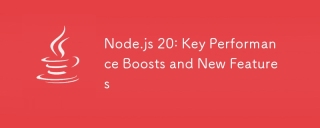
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
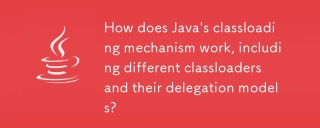
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
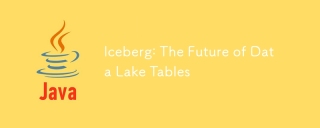
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
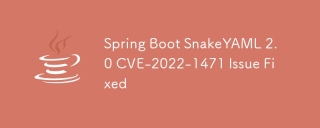
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
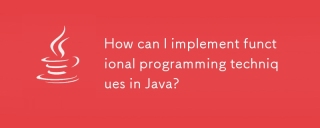
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
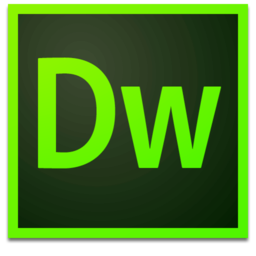
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
