


Rounding Numbers to Significant Figures in Python
When displaying floating-point numbers in a user interface, you often need to round them to a specific number of significant figures. Python offers a convenient way to achieve this using negative numbers.
For integers, you can use negative rounding to specify the number of decimal places:
>>> round(1234, -3) 1000.0
To extend this approach to rounding a float to one significant figure, you can use the following function:
from math import log10, floor def round_to_1(x): return round(x, -int(floor(log10(abs(x)))))
This function calculates the number of decimal places to round based on the absolute value of the number and returns the rounded value.
Here are some examples of how it works:
>>> round_to_1(0.0232) 0.02 >>> round_to_1(1234243) 1000000.0 >>> round_to_1(13) 10.0 >>> round_to_1(4) 4.0 >>> round_to_1(19) 20.0
Note that for floats greater than 1, you may need to convert them to integers before rounding.
The above is the detailed content of How to Round Floating-Point Numbers to One Significant Figure in Python?. For more information, please follow other related articles on the PHP Chinese website!
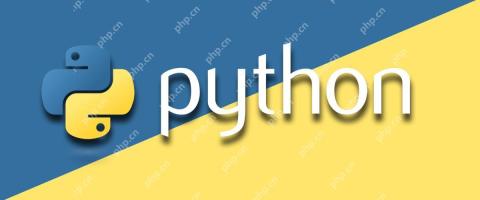
Implementing factory pattern in Python can create different types of objects by creating a unified interface. The specific steps are as follows: 1. Define a basic class and multiple inheritance classes, such as Vehicle, Car, Plane and Train. 2. Create a factory class VehicleFactory and use the create_vehicle method to return the corresponding object instance according to the type parameter. 3. Instantiate the object through the factory class, such as my_car=factory.create_vehicle("car","Tesla"). This pattern improves the scalability and maintainability of the code, but it needs to be paid attention to its complexity
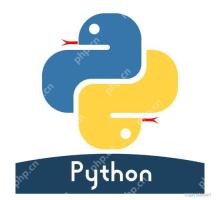
In Python, the r or R prefix is used to define the original string, ignoring all escaped characters, and letting the string be interpreted literally. 1) Applicable to deal with regular expressions and file paths to avoid misunderstandings of escape characters. 2) Not applicable to cases where escaped characters need to be preserved, such as line breaks. Careful checking is required when using it to prevent unexpected output.
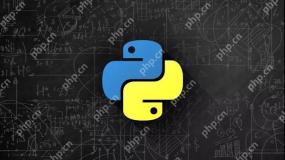
In Python, the __del__ method is an object's destructor, used to clean up resources. 1) Uncertain execution time: Relying on the garbage collection mechanism. 2) Circular reference: It may cause the call to be unable to be promptly and handled using the weakref module. 3) Exception handling: Exception thrown in __del__ may be ignored and captured using the try-except block. 4) Best practices for resource management: It is recommended to use with statements and context managers to manage resources.
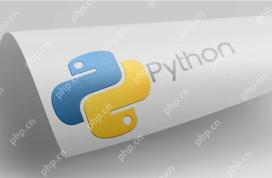
The pop() function is used in Python to remove elements from a list and return a specified position. 1) When the index is not specified, pop() removes and returns the last element of the list by default. 2) When specifying an index, pop() removes and returns the element at the index position. 3) Pay attention to index errors, performance issues, alternative methods and list variability when using it.
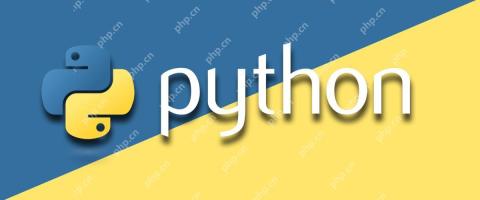
Python mainly uses two major libraries Pillow and OpenCV for image processing. Pillow is suitable for simple image processing, such as adding watermarks, and the code is simple and easy to use; OpenCV is suitable for complex image processing and computer vision, such as edge detection, with superior performance but attention to memory management is required.
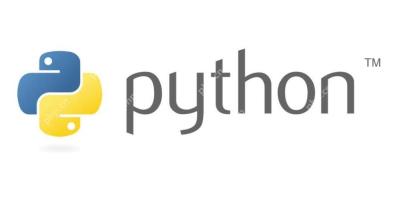
Implementing PCA in Python can be done by writing code manually or using the scikit-learn library. Manually implementing PCA includes the following steps: 1) centralize the data, 2) calculate the covariance matrix, 3) calculate the eigenvalues and eigenvectors, 4) sort and select principal components, and 5) project the data to the new space. Manual implementation helps to understand the algorithm in depth, but scikit-learn provides more convenient features.
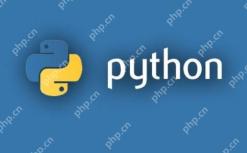
Calculating logarithms in Python is a very simple but interesting thing. Let's start with the most basic question: How to calculate logarithm in Python? Basic method of calculating logarithm in Python The math module of Python provides functions for calculating logarithm. Let's take a simple example: importmath# calculates the natural logarithm (base is e) x=10natural_log=math.log(x)print(f"natural log({x})={natural_log}")# calculates the logarithm with base 10 log_base_10=math.log10(x)pri
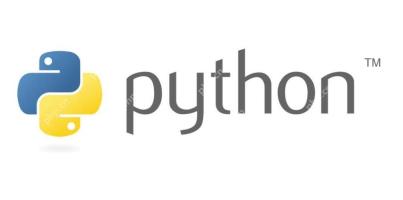
To implement linear regression in Python, we can start from multiple perspectives. This is not just a simple function call, but involves a comprehensive application of statistics, mathematical optimization and machine learning. Let's dive into this process in depth. The most common way to implement linear regression in Python is to use the scikit-learn library, which provides easy and efficient tools. However, if we want to have a deeper understanding of the principles and implementation details of linear regression, we can also write our own linear regression algorithm from scratch. The linear regression implementation of scikit-learn uses scikit-learn to encapsulate the implementation of linear regression, allowing us to easily model and predict. Here is a use sc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
