


How Can You Handle All Possible Exceptions When Using Python's Requests Module?
Handling Exceptions with Python's Requests Module
Catching exceptions when making HTTP requests is crucial for robust error handling. While the provided code snippet can handle some connection-related errors, it misses other potential issues.
According to the Requests documentation, different exception types are raised for:
- Connection errors (including DNS failures and refused connections): ConnectionError
- Invalid HTTP responses: HTTPError
- Timeouts: Timeout
- Excessive redirects: TooManyRedirects
All these exceptions inherit from requests.exceptions.RequestException.
To cover all bases, you can:
- Catch the Base-Class Exception:
try: r = requests.get(url, params={'s': thing}) except requests.exceptions.RequestException as e: # Handle all cases raise SystemExit(e)
- Catch Exceptions Separately:
try: r = requests.get(url, params={'s': thing}) except requests.exceptions.Timeout: # Implement a retry strategy except requests.exceptions.TooManyRedirects: # Notify user of incorrect URL except requests.exceptions.RequestException as e: # Catastrophic error, terminate raise SystemExit(e)
Handling HTTP Errors:
If you need to raise exceptions for HTTP status codes (e.g., 401 Unauthorized), call Response.raise_for_status after making the request.
try: r = requests.get('http://www.google.com/nothere') r.raise_for_status() except requests.exceptions.HTTPError as err: raise SystemExit(err)
By considering all possible exception types and tailoring your error handling strategy, you can ensure your application gracefully handles network issues and provides appropriate responses to users.
The above is the detailed content of How Can You Handle All Possible Exceptions When Using Python's Requests Module?. For more information, please follow other related articles on the PHP Chinese website!
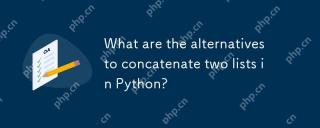
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
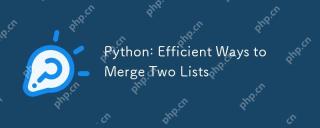
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
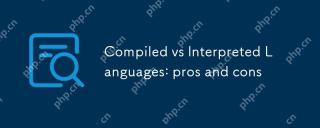
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
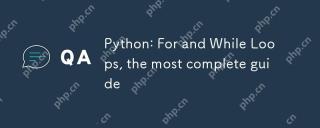
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
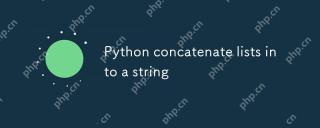
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
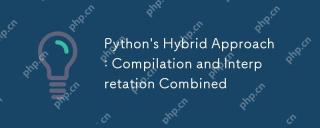
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
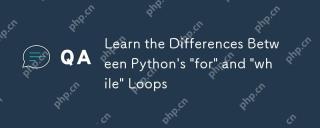
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
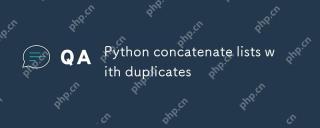
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
