Splitting ArrayLists into Smaller Sublists
In Java, the ArrayList data structure provides a convenient way to store collections of objects efficiently. Sometimes, it becomes necessary to partition an ArrayList into multiple smaller sublists of a specified size. This article explores how to achieve this effectively.
Using subList() for Immutable Views
The subList() method allows you to create views of a portion of an ArrayList. The resulting sublists are immutable, meaning they cannot be modified.
List<integer> numbers = new ArrayList(Arrays.asList(5, 3, 1, 2, 9, 5, 0, 7)); List<integer> head = numbers.subList(0, 4); List<integer> tail = numbers.subList(4, 8);</integer></integer></integer>
After creating the sublists, any changes made to the original ArrayList will be reflected in the sublists. Conversely, modifications to the sublists will not affect the original ArrayList.
Creating Mutable Sublists
If you require mutable sublists, create new ArrayLists from the subList() views.
List<integer> newHead = new ArrayList(head);</integer>
Chopped Function for Convenient Partitioning
For situations where multiple sublists are needed, consider creating a utility function like the following:
static <t> List<list>> chopped(List<t> list, final int L) { List<list>> parts = new ArrayList(); int N = list.size(); for (int i = 0; i (list.subList(i, Math.min(N, i + L)))); } return parts; }</list></t></list></t>
This function takes an ArrayList and a desired sublist length as parameters and returns a list of sublists.
Example Usage
List<integer> numbers = Collections.unmodifiableList(Arrays.asList(5, 3, 1, 2, 9, 5, 0, 7)); List<list>> parts = chopped(numbers, 3); System.out.println(parts); // prints "[[5, 3, 1], [2, 9, 5], [0, 7]]"</list></integer>
Conclusion
By leveraging the subList() method and the utility function discussed in this article, you can effectively split an ArrayList into multiple smaller sublists, tailoring to your specific programming needs.
The above is the detailed content of How to Split an ArrayList into Smaller Sublists in Java?. For more information, please follow other related articles on the PHP Chinese website!
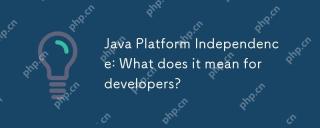
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
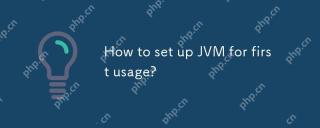
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
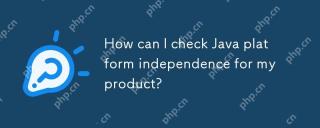
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss
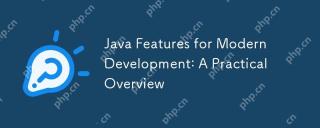
Javastandsoutinmoderndevelopmentduetoitsrobustfeatureslikelambdaexpressions,streams,andenhancedconcurrencysupport.1)Lambdaexpressionssimplifyfunctionalprogramming,makingcodemoreconciseandreadable.2)Streamsenableefficientdataprocessingwithoperationsli
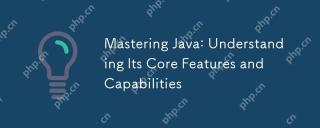
The core features of Java include platform independence, object-oriented design and a rich standard library. 1) Object-oriented design makes the code more flexible and maintainable through polymorphic features. 2) The garbage collection mechanism liberates the memory management burden of developers, but it needs to be optimized to avoid performance problems. 3) The standard library provides powerful tools from collections to networks, but data structures should be selected carefully to keep the code concise.
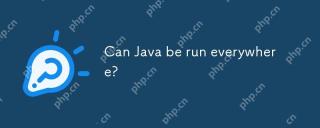
Yes,Javacanruneverywhereduetoits"WriteOnce,RunAnywhere"philosophy.1)Javacodeiscompiledintoplatform-independentbytecode.2)TheJavaVirtualMachine(JVM)interpretsorcompilesthisbytecodeintomachine-specificinstructionsatruntime,allowingthesameJava
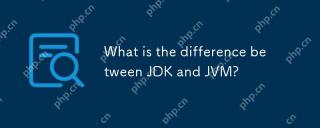
JDKincludestoolsfordevelopingandcompilingJavacode,whileJVMrunsthecompiledbytecode.1)JDKcontainsJRE,compiler,andutilities.2)JVMmanagesbytecodeexecutionandsupports"writeonce,runanywhere."3)UseJDKfordevelopmentandJREforrunningapplications.
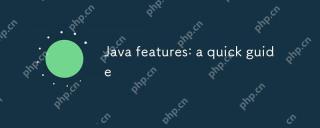
Key features of Java include: 1) object-oriented design, 2) platform independence, 3) garbage collection mechanism, 4) rich libraries and frameworks, 5) concurrency support, 6) exception handling, 7) continuous evolution. These features of Java make it a powerful tool for developing efficient and maintainable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
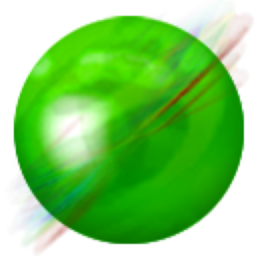
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
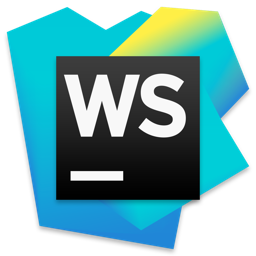
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
