


How does std::forward enable perfect forwarding and preserve the rvalue-ness of arguments?
Understanding std::forward: Its Role in Passing Lvalue and Rvalue References
std::forward is a powerful tool in C for perfect forwarding arguments, ensuring optimal code behavior regardless of whether an lvalue or rvalue reference is passed.
Lvalue vs. Rvalue Intuition
The misconception that "if it has a name, it's an lvalue" can be misleading. While variables generally hold lvalues, this distinction is more about the context and operations involved.
The Need for Perfect Forwarding
Consider a function set that stores a vector v in its data structure _v. The safe approach is to take a reference to v in set, but this may result in an unnecessary copy when v is an rvalue, as in the example below:
set(makeAndFillVector()); // Copies `makeAndFillVector()`'s result into `_v`
Perfect forwarding becomes necessary to preserve the rvalue nature of v and avoid unnecessary copying.
std::forward's Role
std::forward plays a crucial role in完美转发ing arguments by invoking a specific function overload based on the reference type passed. For example:
template<class t> void perfectSet(T &&t) { set(std::forward<t>(t)); }</t></class>
By calling std::forward
Understanding the Behavior
To demonstrate how std::forward preserves the rvalue-ness of an argument, consider the following code:
void perfectSet(T &&t) { set(t); // Preserves `t` as an lvalue set(t); // `t` remains unchanged }
Compare it to:
void perfectSet(T &&t) { set(std::forward<t>(t)); // Preserves `t` as an rvalue set(t); // `t` is now empty due to move semantics }</t>
Without std::forward, the compiler assumes that t might be accessed again and chooses to preserve it as an lvalue. However, using std::forward allows the compiler to correctly handle t as an rvalue, resulting in its contents being moved.
The above is the detailed content of How does std::forward enable perfect forwarding and preserve the rvalue-ness of arguments?. For more information, please follow other related articles on the PHP Chinese website!
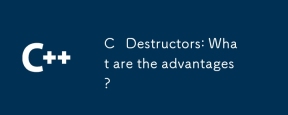
C destructorsprovideseveralkeyadvantages:1)Theymanageresourcesautomatically,preventingleaks;2)Theyenhanceexceptionsafetybyensuringresourcerelease;3)TheyenableRAIIforsaferesourcehandling;4)Virtualdestructorssupportpolymorphiccleanup;5)Theyimprovecode
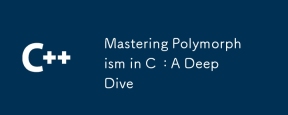
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
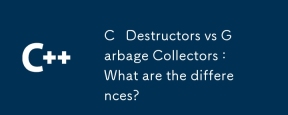
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
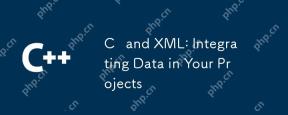
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
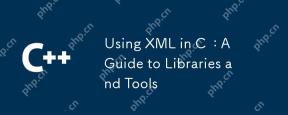
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
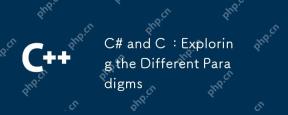
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
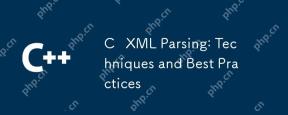
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
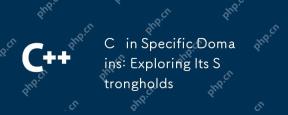
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
