


Conditional INSERT Statements in MySQL: A Custom Approach
Inserting data into MySQL tables is typically straightforward. However, in certain scenarios, you may want to perform conditional inserts based on specific criteria. While stored procedures can handle such tasks, it can be convenient to do so directly in your queries.
A common question arises when dealing with resource allocation. Consider a scenario where you have two tables: products and orders. Orders track products and their quantities, while products maintain the quantity on hand. When an order is placed, you want to insert a new order row only if there is sufficient inventory.
Traditionally, you would check the available quantity using a SELECT statement and then insert the order if the condition is met. However, this approach has concurrency issues. Multiple concurrent orders can read the same quantity on hand before any have been processed, potentially causing overselling.
To address this, you can use a conditional INSERT statement:
INSERT INTO TABLE SELECT value_for_column1, value_for_column2, ... FROM wherever WHERE your_special_condition
In this statement, the INSERT is only performed if the WHERE clause evaluates to true. Using the example schema, you can write a conditional INSERT as follows:
INSERT INTO orders (product_id, qty) SELECT 2, 20 WHERE (SELECT qty_on_hand FROM products WHERE id = 2) > 20;
This statement will only insert an order row if the quantity on hand for product 2 is greater than 20. Otherwise, no rows are inserted, preventing overselling.
This approach combines the functionality of a SELECT statement with the INSERT statement, effectively creating a conditional insert that maintains data integrity even in concurrent scenarios.
The above is the detailed content of How Can You Implement Conditional Inserts in MySQL for Secure Resource Allocation?. For more information, please follow other related articles on the PHP Chinese website!
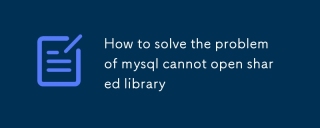
This article addresses MySQL's "unable to open shared library" error. The issue stems from MySQL's inability to locate necessary shared libraries (.so/.dll files). Solutions involve verifying library installation via the system's package m
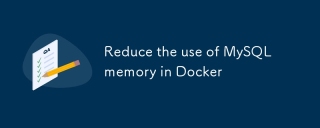
This article explores optimizing MySQL memory usage in Docker. It discusses monitoring techniques (Docker stats, Performance Schema, external tools) and configuration strategies. These include Docker memory limits, swapping, and cgroups, alongside
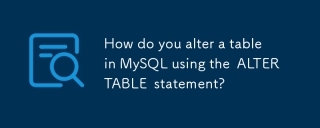
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
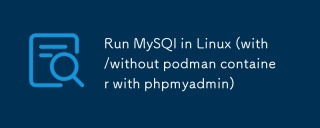
This article compares installing MySQL on Linux directly versus using Podman containers, with/without phpMyAdmin. It details installation steps for each method, emphasizing Podman's advantages in isolation, portability, and reproducibility, but also
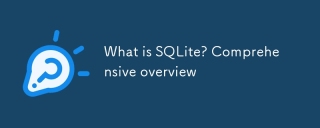
This article provides a comprehensive overview of SQLite, a self-contained, serverless relational database. It details SQLite's advantages (simplicity, portability, ease of use) and disadvantages (concurrency limitations, scalability challenges). C
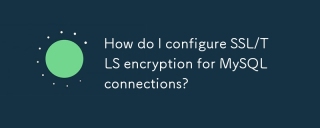
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
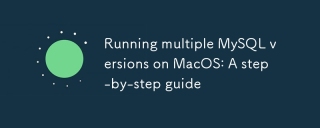
This guide demonstrates installing and managing multiple MySQL versions on macOS using Homebrew. It emphasizes using Homebrew to isolate installations, preventing conflicts. The article details installation, starting/stopping services, and best pra
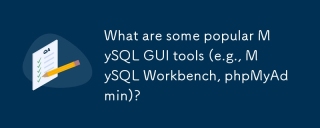
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
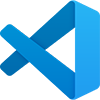
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
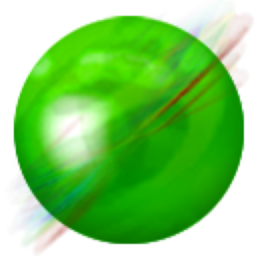
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
