Pinging External IPs from Android Java Applications
When developing a Ping application for Android to verify network connectivity, it's essential to address the limitation of only pinging local IPs. This article will delve into the underlying issue and provide a solution to extend the ping capability to external servers.
The code shared in the original question demonstrates the basic implementation of pinging on Android. However, it falls short when attempting to ping external servers. This is because Android restricts direct network access to hosts outside the device's local network by default.
To overcome this limitation, a workaround involving native system commands is employed. The provided solution leverages Runtime.exec() to execute the /system/bin/ping command with the desired IP address (e.g., 8.8.8.8 for Google DNS).
Here's the updated code incorporating the solution:
private boolean executeCommand(){ System.out.println("executeCommand"); Runtime runtime = Runtime.getRuntime(); try { Process mIpAddrProcess = runtime.exec("/system/bin/ping -c 1 8.8.8.8"); int mExitValue = mIpAddrProcess.waitFor(); System.out.println(" mExitValue "+mExitValue); return mExitValue == 0; } catch (Exception e) { e.printStackTrace(); System.out.println(" Exception:"+e); } return false; }
By utilizing this approach, the executeCommand() method initiates the native ping command, waits for its completion, and returns a boolean indicating the ping result. This enables external servers to be pinged, providing a more comprehensive network connectivity assessment within the Android application.
The above is the detailed content of How to Ping External IPs from Android Java Applications?. For more information, please follow other related articles on the PHP Chinese website!
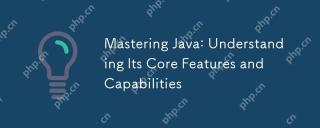
The core features of Java include platform independence, object-oriented design and a rich standard library. 1) Object-oriented design makes the code more flexible and maintainable through polymorphic features. 2) The garbage collection mechanism liberates the memory management burden of developers, but it needs to be optimized to avoid performance problems. 3) The standard library provides powerful tools from collections to networks, but data structures should be selected carefully to keep the code concise.
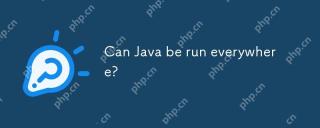
Yes,Javacanruneverywhereduetoits"WriteOnce,RunAnywhere"philosophy.1)Javacodeiscompiledintoplatform-independentbytecode.2)TheJavaVirtualMachine(JVM)interpretsorcompilesthisbytecodeintomachine-specificinstructionsatruntime,allowingthesameJava
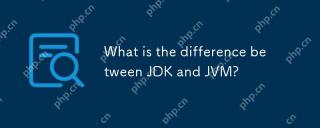
JDKincludestoolsfordevelopingandcompilingJavacode,whileJVMrunsthecompiledbytecode.1)JDKcontainsJRE,compiler,andutilities.2)JVMmanagesbytecodeexecutionandsupports"writeonce,runanywhere."3)UseJDKfordevelopmentandJREforrunningapplications.
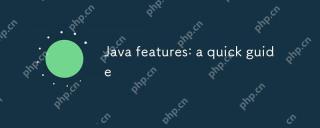
Key features of Java include: 1) object-oriented design, 2) platform independence, 3) garbage collection mechanism, 4) rich libraries and frameworks, 5) concurrency support, 6) exception handling, 7) continuous evolution. These features of Java make it a powerful tool for developing efficient and maintainable software.
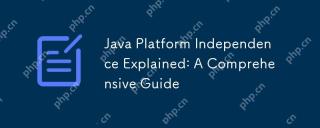
JavaachievesplatformindependencethroughbytecodeandtheJVM.1)Codeiscompiledintobytecode,notmachinecode.2)TheJVMinterpretsbytecodeonanyplatform,ensuring"writeonce,runanywhere."3)Usecross-platformlibraries,becautiouswithnativecode,andtestonmult
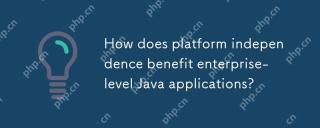
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
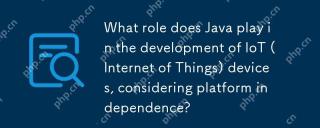
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
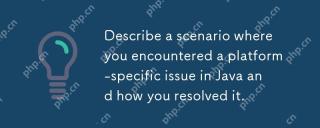
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
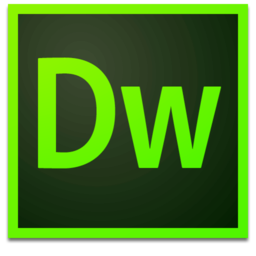
Dreamweaver Mac version
Visual web development tools
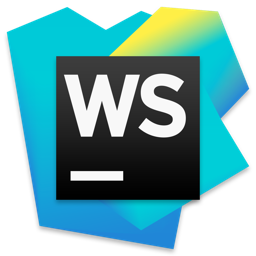
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
