


Merging Multiple Dictionaries into a Single Entity
When dealing with multiple dictionaries, there often arises a need to consolidate them into a single, comprehensive one. This scenario can be encountered in various programming contexts. Let's explore how to merge a list of dictionaries into a single dictionary while handling the potential issue of duplicate keys.
Approach: Iterative Update
To merge a list of dictionaries, you can utilize a straightforward iterative approach. This involves looping through each dictionary in the list and updating an accumulating result dictionary with its contents. Using this method, if multiple dictionaries contain the same key, the value from the latter dictionary will overwrite the existing value in the result dictionary.
result = {} for d in L: result.update(d)
Example:
Given a list of dictionaries:
L = [{'a':1}, {'b':2}, {'c':1}, {'d':2}]
Applying the iterative update approach:
result = {} for d in L: result.update(d)
The resulting dictionary will be:
{'a':1,'c':1,'b':2,'d':2}
Comprehension-Based Approach (Python 2.7 and above)
As an alternative, you can leverage comprehensions to perform the merge operation concisely:
result = {k: v for d in L for k, v in d.items()}
Note:
Keep in mind that dictionaries cannot have duplicate keys. As a result, when merging multiple dictionaries, any duplicate keys will be overwritten by the last corresponding value encountered. If you require the merging of multiple values associated with matching keys, refer to related resources that address this specific scenario.
The above is the detailed content of How to Combine Multiple Dictionaries into a Single Entity in Python?. For more information, please follow other related articles on the PHP Chinese website!
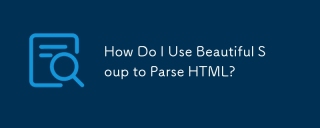
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
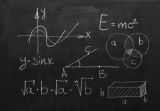
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
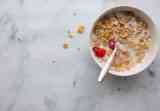
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
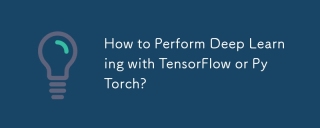
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
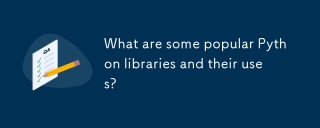
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
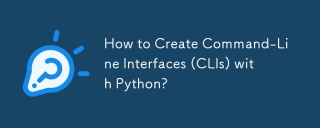
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
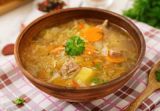
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
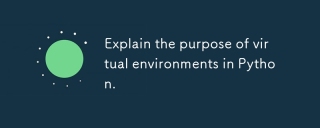
The article discusses the role of virtual environments in Python, focusing on managing project dependencies and avoiding conflicts. It details their creation, activation, and benefits in improving project management and reducing dependency issues.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
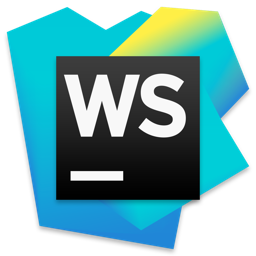
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
