


Android: Resolving ForegroundColorSpan Search Text Highlighting Issue with RecyclerView and ListAdapter
Problem Description
A RecyclerView list of CardViews is located beneath a SearchView within a Toolbar. Search text highlighting with Color.GREEN is intended to be implemented in the ListAdapter's onBindViewHolder() method. However, the first CardView in the RecyclerView list and other subsequent CardViews often fail to display highlighted text, hindering search text visibility. This issue may be linked to using ListAdapter as the Adapter.
Code Snippets
MainActivity
@Override public boolean onCreateOptionsMenu(Menu menu) { mSearchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() { @Override public boolean onQueryTextSubmit(String query) { return false; } @Override public boolean onQueryTextChange(String newText) { filter(newText); return false; } }); return true; } private void filter(String searchText) { ArrayList<card> searchList = new ArrayList(); for (Card cardItem : mCards) { if (cardItem.getTodo().toLowerCase(Locale.US).contains(searchText)) { searchList.add(cardItem); } } if (!searchList.isEmpty()) { adapter.setFilter(searchList, searchText); } }</card>
ListAdapter
public class CardRVAdapter extends ListAdapter<card cardrvadapter.viewholder> { private String searchString = ""; public Spannable spannable; public void setFilter(List<card> newSearchList, String adapSearchText) { if (newSearchList != null && !newSearchList.isEmpty()) { this.searchString = adapSearchText.toLowerCase(Locale.US); ArrayList<card> tempList = new ArrayList(newSearchList); submitList(tempList); } } public class ViewHolder extends RecyclerView.ViewHolder { TextView carBlankText2; // displays text in the CardView and is matched against the search text input. ForegroundColorSpan fcs = new ForegroundColorSpan(Color.GREEN); public ForegroundColorSpan getFCS() { return fcs; } } public ViewHolder(@NonNull final View itemView) { super(itemView); cardBlankText2 = itemView.findViewById(R.id.cardBlankText2); } void bindData(Card card, final int position) { spannable = Spannable.Factory.getInstance().newSpannable(cardBlankText2.getText().toString()); // Get any previous spans and remove them ForegroundColorSpan[] foregroundSpans = spannable.getSpans(0, spannable.length(), ForegroundColorSpan.class); for (ForegroundColorSpan span : foregroundSpans) { spannable.removeSpan(span); } // Highlight matches from search characters is Green color. if (searchString != null && !TextUtils.isEmpty(searchString)) { int index = spannable.toString().toLowerCase(Locale.US).indexOf(searchString); while (index != -1) { spannable.setSpan(getFCS(), index, index + searchString.length(), Spanned.SPAN_EXCLUSIVE_EXCLUSIVE); index = spannable.toString().indexOf(searchString, index + searchString.length()); } cardBlankText2.setText(spannable, TextView.BufferType.SPANNABLE); } } @Override public void onBindViewHolder(@NonNull ViewHolder holder, int position) { final Card card = getCardAt(position); if (card != null) { holder.bindData(card, position); } } public Card getCardAt(int position) { return getItem(position); } }</card></card></card>
Solution
To rectify the highlighting issue, a crucial change must be made to the ListAdapter's setFilter method:
public void setFilter(List<card> newSearchList, String adapSearchText) { if (newSearchList != null && !newSearchList.isEmpty()) { ArrayList<card> tempList = new ArrayList(); for (Card card: newSearchList) { card.setSearchString(adapSearchText.toLowerCase(Locale.US)); tempList.add(card); } submitList(tempList); } }</card></card>
By adding a setSearchString method to the Card model and setting its value when filtering, we trigger a data change that prompts the onBindViewHolder() method to be called, subsequently updating the search text highlighting.
The above is the detailed content of Why is search text highlighting in RecyclerView using ListAdapter failing to display correctly on some CardViews?. For more information, please follow other related articles on the PHP Chinese website!
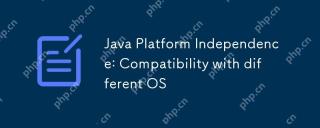
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
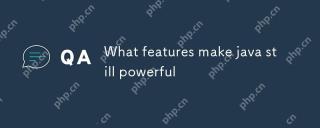
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
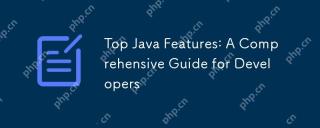
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
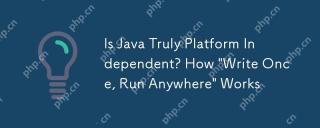
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
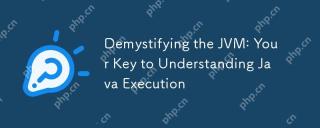
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
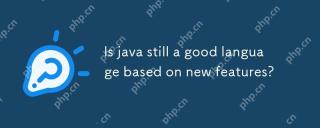
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
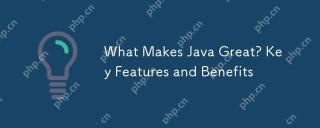
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
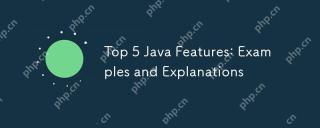
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
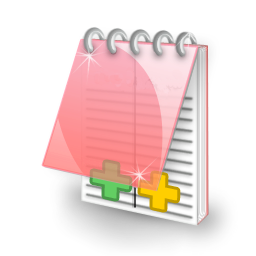
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
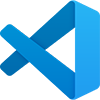
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
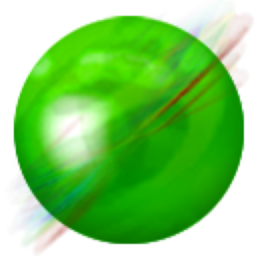
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
