Byte Array to String and Back to Byte Array in Java
Suppose you have an initial byte[] array and need to convert it to a string representation. Subsequently, you want to convert that string back into a byte[] array. This conversion is essential for data transmission and processing in various scenarios.
To convert a byte[] array into a string, you can use the Arrays.toString() method. This method returns a string that represents the content of the byte[] array. However, it's important to note that this string is not a byte[] array anymore. It is a string representation of the byte[] array.
After sending the string over the wire, the receiving end attempts to convert the received string back into a byte[] array. Here is the challenge: the string is no longer a byte[] array; it is a string with a specific format.
To convert the received string back into a byte[] array, you can use the following approach:
- Parse the String: Read the string character by character and extract the numerical value of each byte.
String response = "[-47, 1, 16, 84, 2, 101, 110, 83, 111, 109, 101, 32, 78, 70, 67, 32, 68, 97, 116, 97]"; String[] byteValues = response.substring(1, response.length() - 1).split(",");
- Create Byte Array: Allocate a new byte[] array with the appropriate length.
byte[] bytes = new byte[byteValues.length];
- Assign Byte Values: Loop through each parsed byte value and assign it to the corresponding element in the byte[] array.
for (int i = 0, len = bytes.length; i <ol start="4"><li> <strong>Return Byte Array:</strong> The bytes array contains the original byte data.</li></ol><p>By following this approach, you can effectively convert a byte[] array to a string, send it over the wire, and then convert it back to a byte[] array on the receiving side. This conversion is essential for data manipulation and communication, ensuring that you can work with byte[] arrays throughout the workflow while maintaining the integrity of the data.</p>
The above is the detailed content of How to convert a byte array to a String and back to a byte array in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
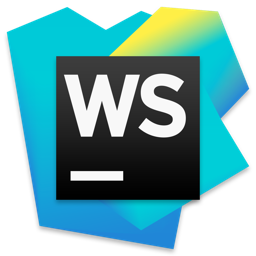
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor