How to Trace Spring RestTemplate Requests and Responses: A Comprehensive Guide
Tracing Spring RestTemplate Requests and Responses: A Comprehensive Guide
Debugging Spring RestTemplate requests and responses can be a perplexing task. This article explores an effective solution to overcome this challenge, providing a comprehensive approach to enable full logging and debugging capabilities.
The Need for Enhanced Logging
In contrast to using curl with the "verbose" option, the Spring RestTemplate often provides limited insights into request and response details. This lack of visibility hinders efficient debugging and troubleshooting.
Customization with ClientHttpRequestInterceptor
To address this issue, Spring Web Client and RestTemplate offer the ClientHttpRequestInterceptor interface. By implementing this interface, developers can customize the behavior of the RestTemplate instance, including the ability to trace both outgoing requests and incoming responses.
A Practical Implementation
To implement the ClientHttpRequestInterceptor, consider the following example:
import org.springframework.http.HttpRequest; import org.springframework.http.client.ClientHttpRequestExecution; import org.springframework.http.client.ClientHttpRequestInterceptor; import org.springframework.http.client.ClientHttpResponse; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import org.slf4j.Logger; import org.slf4j.LoggerFactory; public class LoggingRequestInterceptor implements ClientHttpRequestInterceptor { final static Logger log = LoggerFactory.getLogger(LoggingRequestInterceptor.class); @Override public ClientHttpResponse intercept(HttpRequest request, byte[] body, ClientHttpRequestExecution execution) throws IOException { traceRequest(request, body); ClientHttpResponse response = execution.execute(request, body); traceResponse(response); return response; } private void traceRequest(HttpRequest request, byte[] body) throws IOException { log.info("===========================request begin================================================"); log.debug("URI : {}", request.getURI()); log.debug("Method : {}", request.getMethod()); log.debug("Headers : {}", request.getHeaders() ); log.debug("Request body: {}", new String(body, "UTF-8")); log.info("==========================request end================================================"); } private void traceResponse(ClientHttpResponse response) throws IOException { StringBuilder inputStringBuilder = new StringBuilder(); BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(response.getBody(), "UTF-8")); String line = bufferedReader.readLine(); while (line != null) { inputStringBuilder.append(line); inputStringBuilder.append('\n'); line = bufferedReader.readLine(); } log.info("============================response begin=========================================="); log.debug("Status code : {}", response.getStatusCode()); log.debug("Status text : {}", response.getStatusText()); log.debug("Headers : {}", response.getHeaders()); log.debug("Response body: {}", inputStringBuilder.toString()); log.info("=======================response end================================================="); } }
Using the Interceptor and Buffering
To utilize the interceptor, instantiate the RestTemplate using a BufferingClientHttpRequestFactory and register the LoggingRequestInterceptor as follows:
RestTemplate restTemplate = new RestTemplate(new BufferingClientHttpRequestFactory(new SimpleClientHttpRequestFactory())); List<clienthttprequestinterceptor> interceptors = new ArrayList(); interceptors.add(new LoggingRequestInterceptor()); restTemplate.setInterceptors(interceptors);</clienthttprequestinterceptor>
The BufferingClientHttpRequestFactory is necessary to enable multiple readings of the response body.
Conclusion
By implementing the ClientHttpRequestInterceptor, you can enhance the debugging capabilities of Spring RestTemplate. The example provided in this article demonstrates how to trace both requests and responses, providing comprehensive insights into the communication process. This approach simplifies troubleshooting and improves the efficiency of development and maintenance tasks.
The above is the detailed content of How to Trace Spring RestTemplate Requests and Responses: A Comprehensive Guide. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
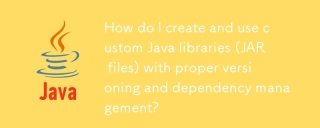
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
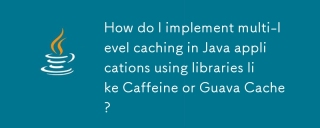
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
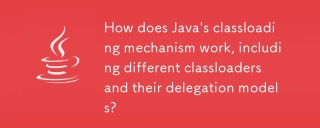
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
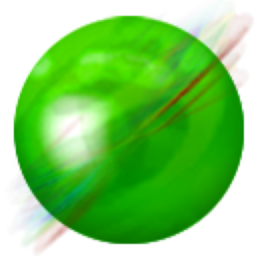
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
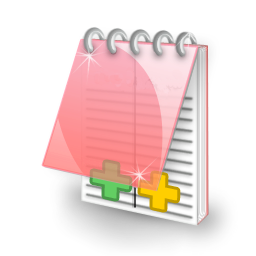
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
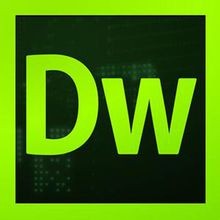
Dreamweaver CS6
Visual web development tools