Checking IP Addresses within a Range Efficiently in Go
Determining whether an IP address falls within a specified range is a common requirement in various network operations. In Go, there are several approaches for addressing this task.
Fastest Method: bytes.Compare
One of the most efficient methods is to use the bytes.Compare function to compare the byte representation of the IP addresses.
import ( "bytes" "net" ) // Check if an IP address is within a range func check(trial, start, end net.IP) bool { if start.To4() == nil || end.To4() == nil || trial.To4() == nil { return false } return bytes.Compare(trial, start) >= 0 && bytes.Compare(trial, end) <p>In this approach, we first check if the given IP addresses are valid IPv4 addresses. We then use bytes.Compare to compare the byte representations of the trial IP and the start and end points of the range. If the comparison results in a non-negative value for both checks, it signifies that the IP address is within the range.</p><p><strong>Example Usage</strong></p><p>The following code demonstrates the usage of the bytes.Compare method:</p><pre class="brush:php;toolbar:false">import ( "fmt" "net" ) var ( ip1 = net.ParseIP("216.14.49.184") ip2 = net.ParseIP("216.14.49.191") ) func main() { check := func(ip string) { trial := net.ParseIP(ip) res := check(trial, ip1, ip2) fmt.Printf("%v is %v within range %v to %v\n", trial, res, ip1, ip2) } check("1.2.3.4") check("216.14.49.185") check("216.14.49.191") }
Output:
1.2.3.4 is false within range 216.14.49.184 to 216.14.49.191 216.14.49.185 is true within range 216.14.49.184 to 216.14.49.191 216.14.49.191 is true within range 216.14.49.184 to 216.14.49.191
The above is the detailed content of How to Efficiently Check if an IP Address is Within a Range in Go?. For more information, please follow other related articles on the PHP Chinese website!
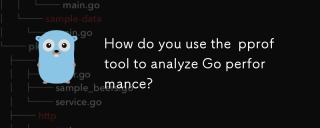
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
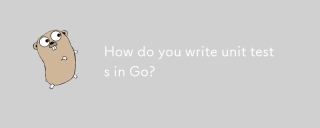
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
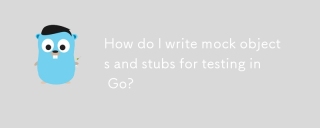
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
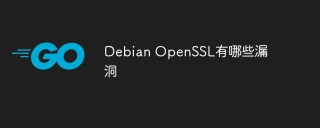
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
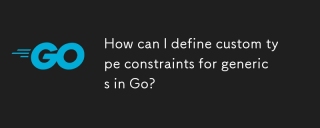
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
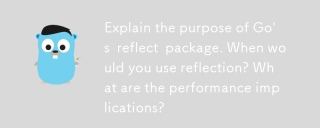
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
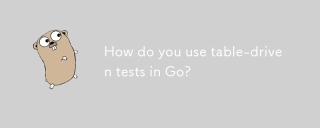
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
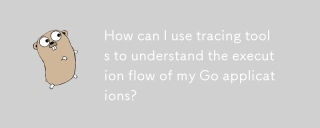
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
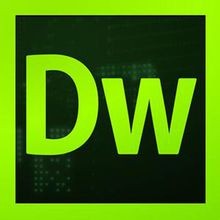
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool