HTTP Basic Authentication in Java using Apache HttpClient
Authenticating with HTTP basic in Java is straightforward. This article demonstrates how to utilize the Apache HttpClient library to implement basic authentication using username and password.
HttpClient 3.0 Implementation
The following code sample illustrates the implementation for HttpClient 3.0:
import org.apache.commons.httpclient.HttpClient; import org.apache.commons.httpclient.auth.AuthScope; import org.apache.commons.httpclient.auth.UsernamePasswordCredentials; import org.apache.commons.httpclient.methods.PostMethod; public class HttpBasicAuth { public static void main(String[] args) { // Create an HTTP client HttpClient client = new HttpClient(); // Set basic authentication credentials client.getState().setCredentials(new AuthScope("ipaddress", 443, "realm"), new UsernamePasswordCredentials("test1", "test1")); // Create a POST method PostMethod post = new PostMethod("http://address/test/login"); // Authenticate using credentials post.setDoAuthentication(true); try { // Execute the POST method and get the response status int status = client.executeMethod(post); System.out.println(status + "\n" + post.getResponseBodyAsString()); } catch (Exception e) { e.printStackTrace(); } finally { // Release connection resources post.releaseConnection(); } } }
HttpClient 4.0.1 Implementation
For HttpClient 4.0.1, here's the corresponding code:
import org.apache.http.HttpEntity; import org.apache.http.HttpResponse; import org.apache.http.auth.AuthScope; import org.apache.http.auth.UsernamePasswordCredentials; import org.apache.http.client.ClientProtocolException; import org.apache.http.client.CredentialsProvider; import org.apache.http.client.methods.HttpPost; import org.apache.http.client.utils.HttpClientUtils; import org.apache.http.impl.client.BasicCredentialsProvider; import org.apache.http.impl.client.DefaultHttpClient; public class HttpBasicAuth { public static void main(String[] args) { try { // Create a default HTTP client DefaultHttpClient httpclient = new DefaultHttpClient(); // Set basic authentication credentials CredentialsProvider credentialsProvider = new BasicCredentialsProvider(); credentialsProvider.setCredentials(new AuthScope(AuthScope.ANY_HOST, AuthScope.ANY_PORT), new UsernamePasswordCredentials("test1", "test1")); httpclient.setCredentialsProvider(credentialsProvider); // Create a POST request HttpPost httppost = new HttpPost("http://host:post/test/login"); // Execute the POST request HttpResponse response = httpclient.execute(httppost); HttpEntity entity = response.getEntity(); // Get the response status and content System.out.println("----------------------------------------"); System.out.println(response.getStatusLine()); if (entity != null) { System.out.println("Response content length: " + entity.getContentLength()); } if (entity != null) { entity.consumeContent(); } // Close the HTTP client HttpClientUtils.closeQuietly(httpclient); } catch (ClientProtocolException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
Enhanced Customization for HttpClient 4.0.1
The provided answer suggests an enhanced implementation for HttpClient 4.0.1 that constructs the "Authorization" header manually:
import java.nio.charset.StandardCharsets; import java.util.Base64; import org.apache.http.HttpHeaders; import org.apache.http.client.methods.HttpPost; // ... // Set basic authentication header String encoding = Base64.getEncoder().encodeToString((user + ":" + pwd).getBytes(StandardCharsets.UTF_8)); httpPost.setHeader(HttpHeaders.AUTHORIZATION, "Basic " + encoding);
The above is the detailed content of How to Implement HTTP Basic Authentication in Java using Apache HttpClient?. For more information, please follow other related articles on the PHP Chinese website!
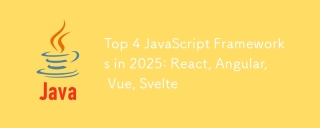
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
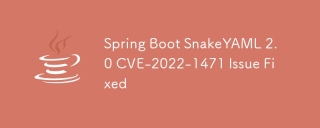
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
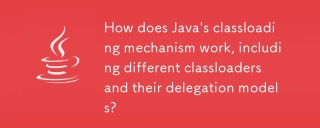
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
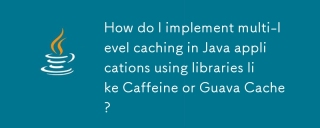
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
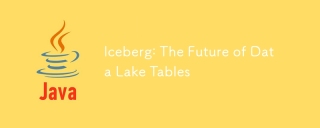
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
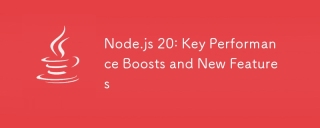
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
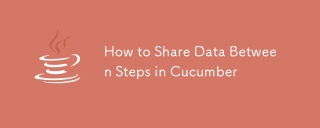
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
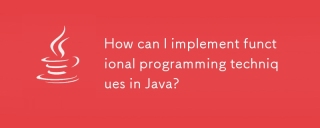
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
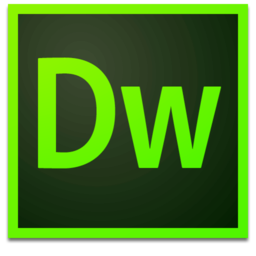
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
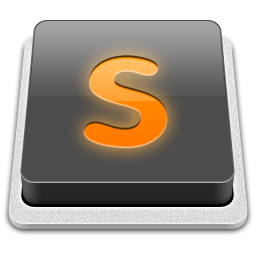
SublimeText3 Mac version
God-level code editing software (SublimeText3)
