Finding the Closest Number in a List to a Given Value
Given a list of integers, you may need to determine which number is closest to a given value. This task can be tackled efficiently using the following methods:
Unsorted List:
If the input list is unsorted, you can utilize the built-in min() function with a key argument. This allows you to find the element with the minimum absolute difference from the target value.
>>> myList = [4, 1, 88, 44, 3] >>> myNumber = 5 >>> min(myList, key=lambda x: abs(x - myNumber)) 4
This method takes O(n) time, as it iterates through the entire list.
Sorted List:
Alternatively, if the list is already sorted or you're willing to sort it once, you can employ the bisection method. This technique uses binary search to locate the insertion point of the target value, effectively finding the closest element in O(log n) time. Here's an example implementation using Python's bisect module:
>>> from bisect import bisect_left >>> myList = sorted([4, 1, 88, 44, 3]) >>> myNumber = 5 >>> bisect_left(myList, myNumber) 2 >>> myList[2] 4
The above is the detailed content of How to Find the Closest Number in a List to a Given Value?. For more information, please follow other related articles on the PHP Chinese website!
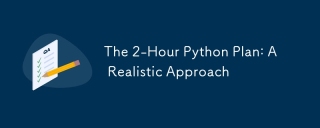
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
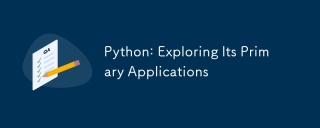
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
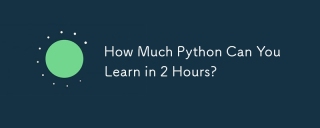
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
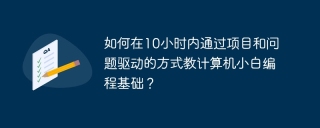
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
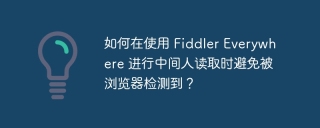
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
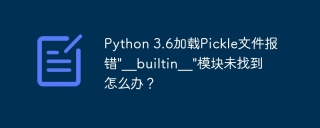
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...
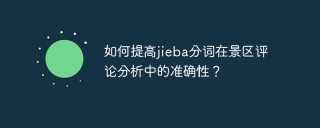
How to solve the problem of Jieba word segmentation in scenic spot comment analysis? When we are conducting scenic spot comments and analysis, we often use the jieba word segmentation tool to process the text...

How to use regular expression to match the first closed tag and stop? When dealing with HTML or other markup languages, regular expressions are often required to...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
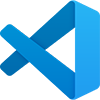
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),